This is study notes of web development involving the relationship and value passing between technologies.
HTML, Hyper Text Markup Language, as its name suggested is a markup language, which does formatting for the document; such as font, border format for cells in table, etc. There are two parts in HTML, head section and body section. Head contains all metadata, controls and the body is the document to be presented in web browser. When URL called by browser, whole HTML file is downloaded in the browser’ computer, which means it is a client side process.
To be easily and programmatically changed or updated, all formats can be centrally managed by a separate file, with extension of .css. This is the file of Cascading Style Sheets, or CSS. The inner link between two files is done through the following declaration to be included in the head of HTML:
‹link rel="stylesheet" type="text/css" href="cssFilename.css"›
In addition, in each division of HTML, “class=”classInCSS”” is needed to point the format class in CSS file. There is no value passing between two files.
Both HTML and CSS do not have programming functionality. To add the programming functionality, JavaScript can be added in HTML. Since programming functions are not of the presentation, it therefore locates in the head of HTML. However, when JavaScript consists too much contents, it can be separated as a file with extension of .js. The inner link between two files is done through the following declaration to be included in the head of HTML:
‹script type='text/javascript' language='Javascript' src='javascriptFileName.js' ›‹/script›
When JavaScript file is called by the HTML in browser’s computer, the whole file is downloaded too. So, JavaScript is also the client side process. Since contents of HTML may need to be changed frequently, separation of HTML and JavaScript may help to improve the refresh speed because programming functions may not change so frequently. This is another reason why JavaScript needs to be an independent file. However, this reason may not sustainable in DHTML scenario, to be mentioned late. At this stage, we deal with the HTML with static contents.
Functions in JavaScript need to be invoked. It can be done through window events, such as mouse move, mouse over, mouse out, mouse click or HTML page on load, etc; or through the calling from another funcation. Since HTML content is static, when value in HTML need to be passed to JavaScript, it is no need to be dealt specifically. Programmer can just use that value when write JavaScript file. In other words, there is no value passed from HTML to JavaScript, apart from invoking action. However, if value passing from JavaScript to HTML is needed, it can be done through “documnt.write()” and “.innerHTML =” methods. “documnt.write()” is located within the body of HTML. Since HTML body is for presentation purpose, to use “documnt.write()” one much first declare it is of JavaScript scripts:
‹script type="text/javascript"›
document.write(' javascriptVariable ');
‹/script›
The major difference between “documnt.write()” and “.innerHTML =” is “documnt.write()” does not need trigger to be active, simply because it is located in the body of HTML. When HTML page loaded, it is invoked. On contrast, “.innerHTML =” is located in JavaScript section/file, it needs to be called either by window events or other functions. Generally, “.innerHTML =” method is recommended. For instance:
document.getElementById('aDivision').innerHTML = javascriptVariable;
By this way, the value javascriptVariable generated from JavaScript functions is delivered to a HTML division called “aDivision”. Since HTML is only the markup language, there will be no variable involved in pure HTML. Variables need to be declared in JavaScript. Indeed, by this way, HTML can have variables. In addition, by this way, the delivered can be not only a value of single variable, but also more complicated contents:
var dynamicTable = ‘‹table›‹tr›‹td›’ + javascriptVariable + ‘‹/td›‹/tr›‹/table›’;
document.getElementById('aDivision').innerHTML = dynamicTable;
Please note, variable dynamicTable now contains some HTML codes, as string. The actual delivered now is no longer the single value but a complete table. This is very much same concept of Dynamic SQL if one is familiar with the database. This indeed is the basic concept of Dynamic HTML, or DHTML, for which the contents together with associated HTML codes are dynamically generated by the running of JavaScript functions.
Each HTML together with its associated CSS and JavaScript files are an independent program. If there are more than one HTML pages in a website, how they are structured? Since HTML and CSS files do not really involve the value passing, Multiple HTML pages can share a single CSS file. One HTML can have more than one JavaScript files by declare both of them in the head:
‹script type='text/javascript' language='Javascript' src='javascriptFile_1.js' ›‹/script›
‹script type='text/javascript' language='Javascript' src='javascriptFile_2.js' ›‹/script›
When a function is called, the browser will first search if “this” file have this function, if not, it would automatically goes to other JavaScript files declared in same HTML file.
It is not recommended to share JavaScript file between HTML pages. Because invoking any function is through window events, such as on page load etc. Sharing JavaScript file just confuses the computer and yourself, on which page load, for instance. Window events such as “onload” is very much HTML page specified and is not generic. However, for those generic functions with absolutely no window events involved, it can be aggregated into a JavaScript file to be shared by multiple HTML files.
Since each HTML page is an independent program, access to other’s functions or passing value between HTML pages is not recommended, for the sake of programming convention as well as security concern. In special cases like one HTML page (child) was opened by another HTML page (parent), it can be done with limitation. For instance:
In parent JavaScript file:
var windowChild = window.open(url); // “url” is the string variable.
windowChild.close();
In this example, parent JavaScript opens a new HTML window and close it immediately.
In child JavaScript file:
var valueFromParent = window.opener.valuePassToChild;
Child HTML can thus access the value of a variable in parent HTML. In case the child window is opened within the iFrame of parent, it is :
var valueFromParent = parent.valuePassToChild;
Please take special note, this syntax is also true for object reference passing through HTML pages. We know that the reference acts as pointer to point to original variable rather than make a copy, any using of the reference, valueFromParent here, is indeed referencing back to parent HTML ‘s object. It does generate the issue of speed. For details, see http://koncordpartners.blogspot.com/. So, please do apply this to the object other than simple variable, such as array.
Following is the method when parent window calls functions located in iFrame child:
document.getElementById('iFrameID').contentWindow
.functionInIFrame(parameter);
If one include this into another function, that function indeed can be called by same or other iFrame windows:
In parent JavaScript file:
function crossWindow(parameter)
{ document.getElementById('iFrameName').contentWindow
.functionInIFrame(parameter);
};
In iFrame file:
crossWindow(parameter);
Unfortunate, the string iFrameName should not be variable, otherwise dynamic function will be involved. Furthermore, dynamic reference passing does never work properly. It therefore dynamic reference and dynamic function should be avoid. In this example, one can use “if” statement to pick up right inner function:
function crossWindow(option, parameter)
{ if (1==option) document.getElementById('iFrameName_1').contentWindow
.functionInIFrame(parameter)
else document.getElementById('iFrameName_2').contentWindow
.functionInIFrame(parameter);
};
When “parameter” is reference to variable located parent window, things become little complex. JavaScript indeed regards it is about the variable in iFrame window. One needs to declare parameter locally first:
var parameter = parent.paraInParent;
What about in iFrame child window, calling corssWindow() function is through dynamic insertion? Something like:
window.onload = function()
{ document.getElementById('dynamicContent').innerHTML = '‹a href="#" onclick="parent.crossWindow(‘ + parameter + ‘)"›Click here to run function in parent window‹/a›';
};
No, it would never work properly if parameter is the reference referring back to another variable in parent window.
(To be continued)
Issues of Array in JavaScript
It is noticed the initialization of a variable when declared as an element of array in not allowed. For instance:
var arr = [1, 2, 3];
var num = arr[0];
Assigning value to variable num "num = arr[0]; " needs to be done separately.
Since JavaScript arrays are assigned by reference, when array to be passed across iFrame, an issue of speed was met, especially for Mozilla Firefox.
Scripts in iFrame page:
var newArray = parent.arr;
When calling newArray[0] immediate after above declaration, it often generates error of "newArray is not defined". HTML pages are actual independent programs (stateless), it is no wonder reference across programs facing such kind of problem. It is therefore suggested avoiding passing array across parent page with iFrame page. Alternatively, slice array into single dimensional variable will do.
The reason behind this issue is this syntax of passing value is also true for object reference passing through HTML pages. We know that the reference acts as pointer to point to original variable rather than make a copy, any using of the reference is indeed reference back to parent HTML's object. It does cause a complex process behind the scent.
For same reason, if one wants to turn array in JavaScript, the best approach is to turn it into a string first, then pass the string to php, then use explode() to turn it back to array in php.
http://www.webdeveloper.com/forum/archive/index.php/t-93920.html
var arr = [1, 2, 3];
var num = arr[0];
Assigning value to variable num "num = arr[0]; " needs to be done separately.
Since JavaScript arrays are assigned by reference, when array to be passed across iFrame, an issue of speed was met, especially for Mozilla Firefox.
Scripts in iFrame page:
var newArray = parent.arr;
When calling newArray[0] immediate after above declaration, it often generates error of "newArray is not defined". HTML pages are actual independent programs (stateless), it is no wonder reference across programs facing such kind of problem. It is therefore suggested avoiding passing array across parent page with iFrame page. Alternatively, slice array into single dimensional variable will do.
The reason behind this issue is this syntax of passing value is also true for object reference passing through HTML pages. We know that the reference acts as pointer to point to original variable rather than make a copy, any using of the reference is indeed reference back to parent HTML's object. It does cause a complex process behind the scent.
For same reason, if one wants to turn array in JavaScript, the best approach is to turn it into a string first, then pass the string to php, then use explode() to turn it back to array in php.
http://www.webdeveloper.com/forum/archive/index.php/t-93920.html
iFrame Height Issue
Changing height of iFrame from JavaScript function becomes painful task in new versions of both IE and Firefox. However, if one uses iFrame, adjusting its height to fit its contents is essential. The problem is the height of content is unknown when dramatic feeding required. The “orthodox” method to accomplish this task is to open iFrame with any height first, then adjust it on onLoad event. Width is less painful because for those iFrame to fit complete screen width, width=“100%” can be defined when iFrame is defined.
Basic idea of this “orthodox” approach includes two steps, detect the correct height and resize to it.
Detecting methods include:
- document.documentElement.clientHeight
- contentWindow.document.body.scrollHeight
Resize method is:
- document.getElementById('myIframe').style.height = newHeight
Basically, this approach does not work. There are several problems around it, such as assignment to .style.height does not work in Firefox. More serious problem is contentWindow.document.body.scrollHeight will return permission denial error message if host page and iFrame content page are not in the same domain; more precisely, the scheme, hostname and port match.
Another approach is to find the correct height before opening the iFrame. It is hard to detect the height of page to be inside of iFrame. However, since iFrame can have its own scroll bar, it may not be necessary to detect content’s height. The best approach might be to find available screen height of user after your title head. Following methods have been tested in Firefox and IE:
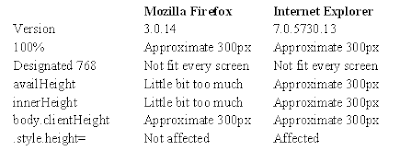
It appears Internet Explorer does not response to any of detections.
Following is the codes to apply this approach:
Codes in head:
‹script type="text/javascript"›
var url = "http://www.google.com/" // URL for your iFrame.
var titleHeight = 240;
var frameHeight = Math.max((screen.availHeight ? (screen.availHeight-titleHeight) : (window.innerHeight ? (window.innerHeight-titleHeight) : document.body.clientHeight)), 768);
window.document.onload = function {document.getElementById('linkIframe').style.height = frameHeight};
‹/script›
Codes in body:
‹script type="text/javascript"›
document.write('‹iframe id="linkIframe" width="100%" height="' + frameHeight + '" src="' + url + '" name="content"›‹/iframe›');
‹/script›
Basic idea of this “orthodox” approach includes two steps, detect the correct height and resize to it.
Detecting methods include:
- document.documentElement.clientHeight
- contentWindow.document.body.scrollHeight
Resize method is:
- document.getElementById('myIframe').style.height = newHeight
Basically, this approach does not work. There are several problems around it, such as assignment to .style.height does not work in Firefox. More serious problem is contentWindow.document.body.scrollHeight will return permission denial error message if host page and iFrame content page are not in the same domain; more precisely, the scheme, hostname and port match.
Another approach is to find the correct height before opening the iFrame. It is hard to detect the height of page to be inside of iFrame. However, since iFrame can have its own scroll bar, it may not be necessary to detect content’s height. The best approach might be to find available screen height of user after your title head. Following methods have been tested in Firefox and IE:
It appears Internet Explorer does not response to any of detections.
Following is the codes to apply this approach:
Codes in head:
‹script type="text/javascript"›
var url = "http://www.google.com/" // URL for your iFrame.
var titleHeight = 240;
var frameHeight = Math.max((screen.availHeight ? (screen.availHeight-titleHeight) : (window.innerHeight ? (window.innerHeight-titleHeight) : document.body.clientHeight)), 768);
window.document.onload = function {document.getElementById('linkIframe').style.height = frameHeight};
‹/script›
Codes in body:
‹script type="text/javascript"›
document.write('‹iframe id="linkIframe" width="100%" height="' + frameHeight + '" src="' + url + '" name="content"›‹/iframe›');
‹/script›
Clearing Obstacles for MS Excel Application Upgrading to 2007
,Recently an Excel application wrote in 2003 version needs to be converted into 2007 following the network infrastructure upgrade. It is noticed there are three parts needed to be attended.
In VBA micro of old application, value of attribute FileFormat for ActiveWorkbook.SaveAs was xlNormal. It is no longer allowed in Excel 2007. The FileFormat now needs to be explicitly expressed. Common values are:
51 = xlOpenXMLWorkbook (without macro's in 2007, xlsx)
52 = xlOpenXMLWorkbookMacroEnabled (with or without macro's in 2007, xlsm)
50 = xlExcel12 (Excel Binary Workbook in 2007 with or without macro's, xlsb)
56 = xlExcel8 (97-2003 format in Excel 2007, xls)
In this case, value of 52 is used. For more information, please refer to http://www.rondebruin.nl/saveas.htm.
File name is also an issue. This application automatically archives data everyday into a file, of which there is a space in file’s name. Now VBA in Excel 2007 converted the space to ‘%’ sign. Accordingly, the archiving file’s name needs to be changed, as well as the file type changed from .xls to .xlsm.
Originally archiving function involved large chunk of cells being copied from one worksheet to another. After conversion to Excel 2007, it is noticed the file’s size increase drastically without apparent reason. The educated guessing is somehow the application accumulated its clipboard during the automatic archiving process which involved copy-paste and save-as. However, nothing was found either in worksheets or clipboard. Web search shows no such error had been reported to Microsoft. After rewrote codes in this part to remove format as part of clipboard, the problem is resolved, although the reason is still not be found.
In VBA micro of old application, value of attribute FileFormat for ActiveWorkbook.SaveAs was xlNormal. It is no longer allowed in Excel 2007. The FileFormat now needs to be explicitly expressed. Common values are:
51 = xlOpenXMLWorkbook (without macro's in 2007, xlsx)
52 = xlOpenXMLWorkbookMacroEnabled (with or without macro's in 2007, xlsm)
50 = xlExcel12 (Excel Binary Workbook in 2007 with or without macro's, xlsb)
56 = xlExcel8 (97-2003 format in Excel 2007, xls)
In this case, value of 52 is used. For more information, please refer to http://www.rondebruin.nl/saveas.htm.
File name is also an issue. This application automatically archives data everyday into a file, of which there is a space in file’s name. Now VBA in Excel 2007 converted the space to ‘%’ sign. Accordingly, the archiving file’s name needs to be changed, as well as the file type changed from .xls to .xlsm.
Originally archiving function involved large chunk of cells being copied from one worksheet to another. After conversion to Excel 2007, it is noticed the file’s size increase drastically without apparent reason. The educated guessing is somehow the application accumulated its clipboard during the automatic archiving process which involved copy-paste and save-as. However, nothing was found either in worksheets or clipboard. Web search shows no such error had been reported to Microsoft. After rewrote codes in this part to remove format as part of clipboard, the problem is resolved, although the reason is still not be found.
Subscribe to:
Posts (Atom)
Labels
- :: (1)
- ? (1)
- .bat (1)
- .css (1)
- .getElementById (1)
- .htaccess (2)
- .html (1)
- .iso (3)
- .js (2)
- .js.php (2)
- .length (1)
- .parent (1)
- .php (1)
- .replace() (3)
- .replace(RegExp) (2)
- .search() (2)
- .SendMail (1)
- .sql (1)
- .style.height (1)
- .write (1)
- 'N' (1)
- 'null' (1)
- 'title' (1)
- 'undefined' (2)
- "Canvas" (1)
- "top()" (1)
- ( (1)
- () (1)
- (a) (1)
- (a)count() (1)
- [ (1)
- [...] (1)
- [0] (1)
- [rsInvalidDataSetName] The table ‘table1’ refers to an invalid DataSetName (1)
- { (1)
- * (1)
- \ (1)
- \n (2)
- \t (1)
- % (2)
- %...% (1)
- ^ (1)
- + (1)
- | (1)
- $ (1)
- $end (1)
- $this- (1)
- 0 (1)
- 1 OR -1 (1)
- 1280x1024 (1)
- 1680x1050 (1)
- 1920x1200 (1)
- 1σ (1)
- 2560x1600 (1)
- 32-bit (2)
- 34.1% (1)
- 3rd Normal Form (1)
- 64-bit (2)
- 7680x4800 (1)
- a (2)
- Access (1)
- Across Different Rows (1)
- Across HTML Pages (1)
- Action Query (1)
- Active (1)
- ActiveWorkbook (1)
- ADD COLUMN (1)
- Address (1)
- ADDRESS() (1)
- AdSense (1)
- Advanced Editing Toolbar (1)
- Aggragate function (1)
- AJAX (2)
- Algorithm (1)
- ALTER TABLE (1)
- Analytic Functions (1)
- Anchor (1)
- Annualized Projection (1)
- Anonymous Function (1)
- Another Table (1)
- ANSI SQL (1)
- Append Array Into Another Array (1)
- ArcCatalog (1)
- ArcEditor (2)
- ArcGIS (1)
- ArcMap (1)
- Arithmetic Mean (1)
- Array (6)
- Array Data Type (1)
- Array Slice (1)
- Array Type (1)
- array_merge() (1)
- array_push() (1)
- Artificial Intelligence (1)
- ASCII (1)
- ASCII Key Code (1)
- ASPX (1)
- Assembly (1)
- Associative Array (3)
- Attribute (2)
- Atul Kumar (1)
- Auto-Number (1)
- AUTOINCREMENT (1)
- Automatic (1)
- Automatic Login (1)
- Automatically Added Element (1)
- Automation (3)
- availHeight (1)
- AVG() (1)
- Aviation (1)
- Background Image (1)
- Batch File (1)
- bcc (1)
- Best Length (1)
- BI (2)
- Big5 (1)
- Bind Variable (5)
- blog.dwnews.com (1)
- Bookmarks (1)
- Boolean (1)
- Bracket (4)
- Bracket () (1)
- Browser (1)
- Bug (2)
- Bulk (1)
- Bulk Collect (1)
- Business Intelligence (2)
- Button (1)
- C# (1)
- c2coff (1)
- Calculation (1)
- Calendar Control (1)
- Caps Lock (1)
- CASE (5)
- CAST (1)
- cc (1)
- CD/DVD (1)
- CDO (1)
- CEIL (1)
- Cell (3)
- Charset (1)
- Checkbox (1)
- Chinese Characters (2)
- Chute Time (1)
- Circle (1)
- Class (2)
- Class Selector (1)
- Clean Code (1)
- Clean Computer (1)
- clientHeight (1)
- Clipboard (1)
- Closing Identifier (1)
- Closures (1)
- Code Editor (1)
- Code Cleaning (3)
- Code Cleanser (1)
- Code Compress (1)
- Code Compression (1)
- Code Compressor (1)
- Code Conventions (4)
- Code Optimization (1)
- Code Optimizer (1)
- Collection (1)
- Color Code in HTML (1)
- Column Alias (1)
- Column Name (3)
- Comma (1)
- Comments (2)
- Compact and Repair (1)
- Comparison (1)
- Comparison of IF Statement (1)
- Computer Science (1)
- Concatenation (1)
- Condition Set (1)
- Conditions (1)
- contentWindow (1)
- Convert (1)
- Convert String To Reference (1)
- CONVERT() (4)
- Coordinates Parse (1)
- Copy (3)
- count() (1)
- Create (1)
- Create Table (1)
- CREATE VIEW (1)
- Crimson Editor (1)
- Cross Join (1)
- Cross Windows (1)
- Crystal Reports (2)
- Crystal X (1)
- Crystal XI (1)
- CSS (4)
- Ctri+Shit+Enter (1)
- cx (1)
- Data Connection Wizard (1)
- Data Layout (1)
- Data Layout in Report (1)
- Data Type (2)
- Data Warehouse (1)
- Database (3)
- Dataset (2)
- DataSetName (1)
- Datatype (1)
- Date Format (2)
- DATEADD() (1)
- DATEDIFF() (1)
- DATEPART() (1)
- Dates Inclusive (1)
- Days in Month (1)
- DBA (1)
- Debug (1)
- Decimal Place (2)
- Decimal Point (2)
- DECIMAL() (1)
- DECODE (1)
- Default Database (1)
- Delegate (1)
- DELETE (3)
- Deleted Records (1)
- Delimited By Comma (1)
- Denormalized Data Structure (1)
- Deprecation (1)
- Description (1)
- DHTML (8)
- Dialogue Window (1)
- Different Servers (1)
- DISABLE CONSTRAINT (1)
- Disk Image (1)
- Disk Space (1)
- Disorderly Sorting Criterion (1)
- DISTINCT (1)
- Distributed Computing (1)
- DIV (2)
- DO (1)
- document.body.appendChild() (1)
- document.body.clientHeight Does Not Work (1)
- document.body.clientWidth/Height (1)
- document.body.offsetWidth/Height (1)
- document.createElement() (1)
- document.documentElement.clientWidth/Height (1)
- document.getElementById().innerHTML (2)
- document.getElementById().value (1)
- document.write() (3)
- Does Not Calculate (1)
- DOM (1)
- domain (1)
- Don Burleson (1)
- Double Quote (1)
- Drag and Drop (1)
- Draw Circle (1)
- DROP (1)
- Drop Down List (1)
- DSN (1)
- Dump Tests (1)
- Dynamic (2)
- Dynamic Codes (2)
- Dynamic Column Name (1)
- Dynamic Column Numbers (2)
- Dynamic Columns (1)
- Dynamic Dimension (1)
- Dynamic HTML (7)
- Dynamic Query (3)
- Dynamic SQL (2)
- Dynamic Table Name (1)
- Element (1)
- Embed (2)
- Empty String (1)
- empty() (1)
- ENABLE CONSTRAINT (1)
- Enable PHP (3)
- Encapsulation (2)
- End Bracket (2)
- End Tag (2)
- Enforce Width (1)
- Equivalent of window.innerWidth/Height (1)
- Error (2)
- Error Console (1)
- Error Massage (1)
- Error Message (10)
- Error message: Operation must use an updateable query (1)
- Error Number 2950 (1)
- Error: Function expected (1)
- Error: Invalid argument (1)
- Error: is not a function (1)
- Escape (1)
- Escape Sequence (2)
- eval() (1)
- Event (2)
- Examination (1)
- Exams (1)
- Excel (6)
- Excel 2003 (1)
- Excel 2007 (1)
- Excel Functions (1)
- EXEC (3)
- EXEC sp_executesql (1)
- EXEC() (1)
- EXECUTE (2)
- EXECUTE() (1)
- Existing (1)
- Existing Table (1)
- Explain Plan (1)
- explode() (1)
- External Data (1)
- FALSE (1)
- Fast (1)
- Fatal error: Call to undefined function... (1)
- Favorites (1)
- FileFormat (1)
- Firefox (3)
- First Day in Week (1)
- First Element (1)
- First Week in Month (1)
- First Week in Year (1)
- Fiscal Year (1)
- Flag (1)
- Float (1)
- FLOOR (1)
- for...in (1)
- Force Download (1)
- Force Update (1)
- Forecast (1)
- Form (6)
- Format (2)
- Format Cells (1)
- Formula (2)
- Formula Shown Up (1)
- Friday (1)
- Function (2)
- Function Declaration (2)
- Function Literal (4)
- Function Object (1)
- Function Passing (2)
- Function Pointer (3)
- Function Reference (3)
- GB (1)
- GB18030 (2)
- GB2312 (1)
- GB2312-80 (1)
- General (1)
- Geodata (2)
- getElementId() (1)
- GIS (3)
- Global Temprary Table (1)
- Google (4)
- Google Maps (1)
- GROUP BY (3)
- GTT (1)
- Handwriting (1)
- Hardware Engineering (1)
- header() (1)
- Heredoc (1)
- Hexadecimal (1)
- Hierarchy (1)
- Historic Data (1)
- hl (1)
- Homepage (2)
- Horizontal (1)
- Hour (1)
- Hover (1)
- Howard Stone (1)
- href= (1)
- HTML (20)
- HTML Color Code (1)
- HTML Loading Sequence (1)
- HTML Shows Nothing (1)
- HTML Table (1)
- http (1)
- HVM (1)
- IA64 (1)
- IDE (1)
- Identifier (1)
- Identifier URL (1)
- Identify (1)
- ie (6)
- IE 8 (1)
- IE Bug (2)
- IF (1)
- IF ELSE (1)
- IF ELSE Statement (2)
- IF Statement (1)
- if() (1)
- iFrame (3)
- iFrame Height (1)
- IIF (1)
- IIF() (1)
- Image (1)
- Import and Export Data (32-bit) (1)
- Importing Identifier (1)
- IN (1)
- Include (1)
- Indent (1)
- Indentation (3)
- Index (2)
- Indexed Array (2)
- INDIRECT() (1)
- Information Management (1)
- Information Science (1)
- Information Technology (1)
- Inheritance (1)
- INNER JOIN (2)
- Inner Query (2)
- innerHeight (1)
- Input (1)
- Input Item (1)
- Insert (2)
- Installer Structure (1)
- Instantiation (1)
- INT (1)
- Integer (1)
- Interface (2)
- Internet Explorer (4)
- Internet Explorer 8 (1)
- Interquartile Mean (2)
- Intersection (1)
- Invalid Argument (1)
- IQM (3)
- is not a function (1)
- IS NULL (1)
- Is Number (1)
- Is Numeric (1)
- is_float() (1)
- is_int() (2)
- is_null() (1)
- is_numeric() (3)
- Is_numeric() 0 (1)
- is_string() (1)
- isNumber (1)
- ISNUMBER() (1)
- ISO 8601 (1)
- iso Date Format (1)
- iso Format (3)
- ISO Image (3)
- isset() (1)
- IT (1)
- IT Certification (1)
- IT Exames (1)
- Itzik Ben-Gan (1)
- Japanese (1)
- Japanese Characters (1)
- Java (3)
- JavaScript (35)
- JavaScript Array (3)
- JavaScript Block (1)
- JavaScript Debug (1)
- JavaScript Download (1)
- JavaScript Event (1)
- JavaScript File (1)
- JavaScript File Download (1)
- Javascript File Generated by PHP (1)
- JavaScript Key Code (1)
- JavaScript Keycode (1)
- Javascript to PHP (1)
- JeSO (1)
- Job (2)
- Join (1)
- JS (1)
- JSON (3)
- JSON Format (1)
- Ken Stevens (2)
- Key (4)
- Key Word (1)
- Key-only Array (1)
- Keyword (2)
- Koncord (3)
- Koncord Applied Excel Functions (2)
- Koncord Cleanser (1)
- Koncord Homepage (2)
- Korean (1)
- Korean Characters (1)
- Lambda Expression (1)
- Landscape (1)
- lang_zh_Hans (1)
- lang_zh_Hant (1)
- Language (3)
- Languages (1)
- Large Array (1)
- Last Weekday (1)
- last_day (2)
- Latitude (2)
- Leap Year (1)
- Length (3)
- Line Break (1)
- Linear String (1)
- Link (2)
- Linked Server (1)
- Linux (1)
- ListBox (1)
- Literal (3)
- Loading (1)
- Local Address (1)
- Logic (1)
- Logic Bug (2)
- Logic Error (2)
- Long URL (1)
- Longitude (2)
- Loop Statement (1)
- LPAD (1)
- lr (1)
- Machine read (1)
- Macro (1)
- Macros (1)
- Marker (1)
- Match (2)
- Mathematics (1)
- Max (1)
- Max Length (3)
- Max Size (1)
- MAX() (1)
- Mean (1)
- Median (1)
- Megapixels (1)
- Memory (1)
- meta (1)
- Method (1)
- Micro (1)
- Microsoft Access (2)
- Microsoft Bug (1)
- Microsoft Excel (1)
- Microsoft Office Access (1)
- Microsoft Visual Studio 2005 (1)
- Microsoft Visual Studio 2008 (1)
- Military Time (1)
- Minute (1)
- Missing Hard Disk Space (1)
- mod_rewrite (2)
- Modular Programming (1)
- Modules (2)
- Monday (1)
- Monitor (1)
- Move (2)
- MS Access (13)
- MS Access 2000 (1)
- MS Access 2007 (1)
- Ms Excel (1)
- Multi-statement Table-Valued Function (1)
- Multidimensional Array (5)
- Multiple Email Recipients (1)
- Multiple Parameters (1)
- Multiple Recipients (1)
- Multiple-Value Parameter (1)
- multiple-value parameters (1)
- MySQL (5)
- MySQL 5.1 (1)
- MySQL Query (1)
- Name (1)
- Namespace (1)
- NaN (1)
- NCHAR (1)
- Nested Array (1)
- Nested Functions (1)
- Nested Object Namespacing (1)
- New (1)
- Newline (2)
- No Selection (1)
- non-fatal error (1)
- Normal Form (1)
- Normally Distributed Data (1)
- not a function (1)
- NOT IN (1)
- Notepad (2)
- Nothing (1)
- Nowdoc (1)
- NTEXT (1)
- Null (5)
- Number (1)
- Number 0 (1)
- Number of Elements (1)
- Numeric (2)
- Numerical Data Type (2)
- NVARCHAR (2)
- Object (3)
- Object Oriented (1)
- Object-Oriented (1)
- ODBC (2)
- OLAP (1)
- OLAP Database (1)
- OLTP (1)
- onChange (1)
- One-stroke Handwriting (1)
- onkeydown (1)
- onkeypress (1)
- Online (1)
- onload event (2)
- OO (1)
- OpenID (1)
- OpenID 1.1 (1)
- Operation must use an updateable query (1)
- Option (1)
- Option List (2)
- Optional Parameters (1)
- optionSelected (1)
- Oracle (7)
- Oracle Application Patch (1)
- Oracle Enterprise Linux (4)
- Oracle Procedure (1)
- Oracle VM (1)
- Oracle VM Template (1)
- Oracle XML Function (1)
- ORDER BY (3)
- Outer Join (1)
- Outer Query (1)
- OUTPUT (2)
- OVER PARTITION BY (1)
- Override Order (2)
- Parameter (7)
- Parameter Management (2)
- Parameter Sequence (1)
- Parameters (2)
- Parentheses () (1)
- Parse Error (1)
- Parsing Inside (1)
- PARTITION (1)
- Passing Array (1)
- Passing Function (2)
- Passing Name (1)
- Passing Reference (1)
- Passing Value (1)
- Passing Value iFrame (1)
- Paste (2)
- Paste Values (1)
- Patch (1)
- Percent (2)
- Percentage (1)
- Percentage Format (1)
- Performance (1)
- Performane Tuning (2)
- Permission (1)
- Peter Michaux (1)
- PHP (19)
- PHP Array (2)
- PHP Tag (1)
- PHP Wrapping JavaScript Debugging Method (2)
- phpinfo() (1)
- PIVOT (4)
- Pixel (1)
- PL/SQL (8)
- Portrait (1)
- Precise Radius (1)
- Prefix 'N' (1)
- Preselected (1)
- Privacy (1)
- Private (3)
- Private Search Engine (2)
- Probability Distribution (1)
- Procedure (1)
- Progress Bar (1)
- Project (1)
- Projection (1)
- Protected (1)
- Prototype (1)
- Public (3)
- public_html (1)
- Publisher ID (1)
- Pure Code Editor (1)
- push() (1)
- PV (1)
- q (1)
- Q and A (5)
- qmchenry (1)
- Radius (1)
- Random Access (1)
- Reconstruct Function (1)
- Recover (1)
- Recovery (1)
- Redirect (2)
- ref cursor (1)
- Reference (2)
- Reference Instantiate (1)
- RegExp (4)
- Regular (1)
- Regular Expression (1)
- Remote Server (1)
- Removal (1)
- Remove (1)
- Report (6)
- Reporting (1)
- Reporting Services (2)
- Reporting Services Database (1)
- ReportingServices.js (1)
- Require (1)
- Resolution (1)
- RewriteEngine (1)
- RewriteOptions (1)
- RewriteRule (1)
- Rizal Almashoor (1)
- Robotics (1)
- Ron de Bruin (1)
- ROUND() (2)
- ROUNDDOWN() (1)
- Rounding (1)
- Row (1)
- Row to Column (1)
- ROWNUM (1)
- sa (1)
- Saturday (1)
- Save As (1)
- SaveAs (1)
- Schedule (2)
- Screen Size (1)
- Script (1)
- Script File (2)
- scrollHeight (1)
- Search (2)
- Search Engine (4)
- Seasonal Adjustment (1)
- Secret Process (1)
- SELECT (4)
- Select List (1)
- SELECT PIVOT (2)
- SELECT TOP (1)
- Selected Item (1)
- selectedIndex (1)
- Selector (1)
- Self JOIN (1)
- Self-ting Temporary Function (1)
- self:: (1)
- SEO (2)
- Sequence (3)
- Sequence of Parameters (1)
- Sequence to Execute Modules (2)
- Sequential Number (1)
- Sequential Programming (1)
- Series Number (1)
- Server Virtualization (1)
- set (4)
- SET NAMES (1)
- SETI(a)home (1)
- setInterval (2)
- setTimeout (1)
- SetWarnings (1)
- Shared Server (1)
- Show/Hide (1)
- SHP (1)
- sign() (1)
- Simplified Chinese (2)
- SIZE (1)
- sizeof() (1)
- Slow Computer (1)
- Smifis (1)
- Software Engineering (1)
- Solution (1)
- Sort (1)
- Sorting (2)
- Sorting Order (1)
- SP (1)
- sp_executesql (2)
- Space (1)
- SPAN (1)
- Specific Radius (1)
- Speed (1)
- sq_addlinkedserver (1)
- sq_addlinkedsrvlogin (1)
- SQL (5)
- SQL Editor (1)
- SQL Query (1)
- SQL Server (12)
- SQL Server Agent (1)
- SQL Server Analysis Services (1)
- SQL Server Business Intelligence Development Studio (1)
- SQL Server Integration Services (1)
- SQL Server Management Studio (2)
- SQL Server Native Client 10.0 (2)
- SQL Server Reporting Services (6)
- SQL Server Reporting Services 2005 (2)
- SSAS (1)
- SSIS (1)
- SSRS (7)
- SSRS 2005 (3)
- SSRS 2008 (2)
- SSRS Parameter (1)
- Standard Deviation (2)
- Startup (1)
- Stateless (1)
- Static (2)
- Status Bar (1)
- STD() (1)
- STDDEV() (1)
- STDEVP() (1)
- Stored Procedure (7)
- String (6)
- String 'null' (1)
- String 0 (1)
- String Parse (1)
- String Reference (2)
- Stringify (1)
- stringify() (1)
- strlen() (1)
- Style Properties (1)
- subdomain (1)
- SUBSTRING (1)
- SUM() (2)
- SUM(CASE) GROUP BY Method (3)
- Summation (1)
- Summation of Hours (1)
- Sunday (1)
- Suppress (1)
- T-SQL (26)
- Tab (2)
- Table (3)
- Table Name (2)
- Table of Contents (1)
- Table Structure (1)
- Table Type (1)
- Table() (1)
- Task Manager (1)
- td (2)
- Telephone Number Parse (1)
- Temp Table (2)
- Temporary Table (2)
- Terms (1)
- Terms Of Services (1)
- Test Books (1)
- Text (1)
- The report definition is not valid (1)
- The SELECT item identified by the ORDER BY number 1 contains a variable as part of the expression identifying a (1)
- this (2)
- Thursday (1)
- Tin() (1)
- TINYINT (1)
- Tinyint() (1)
- Title (1)
- To_number() (1)
- Today() (1)
- Tool (1)
- Toolbar (1)
- TOP (1)
- TOP (n) PERCENT (1)
- Total (1)
- tr (1)
- Traditional Chinese (2)
- Transact-SQL (2)
- TRANSFORM (4)
- Transpose (4)
- trim() (1)
- TRUE (1)
- Truncate (2)
- Tuesday (1)
- Tutorial (7)
- typeof() (1)
- undefined() (1)
- UNDELETE (1)
- UNDO (1)
- Uneven Array (1)
- Unexpected $end (1)
- Unicode (2)
- Unicode 3.0 (1)
- UNION (5)
- UNION ALL (1)
- Unknown Dimentions (1)
- Unneeded Parameters (1)
- unset() (2)
- Unwanted Parameters (1)
- Update (2)
- Upload (1)
- Upload Data (1)
- Upper letters (1)
- Urban Legend (1)
- URL (1)
- URL Redirect (2)
- Usability (1)
- use Varibalized Function (1)
- UTF-16 (1)
- UTF-8 (1)
- UTF8 (1)
- Value (2)
- Value Passing (1)
- var (1)
- VARCHAR (1)
- Varchar To Integer (1)
- VARCHAR(MAX) (2)
- Variable Assignment (1)
- Variable Declaration (1)
- Variable Passing (2)
- Variablized Function (4)
- VB6 (2)
- VBA (3)
- VDS (1)
- Vertical (1)
- Virtual Dedicated Server (1)
- Virtual Private Server (1)
- Virtual URL (1)
- Visited (1)
- Visual Basic 6.0 (2)
- Visual Studio (1)
- VMware (1)
- VMware Server (2)
- VPS (1)
- WebSearch (1)
- Wednesday (1)
- Week Start Day (1)
- WEEKDAY (1)
- WEEKDAY() (2)
- WHERE (2)
- WHERE Condition (3)
- WHERE IN (1)
- WHERE NOT IN (1)
- Whitespace (2)
- WHUXGA (1)
- Width (1)
- window.innerWidth (1)
- window.onload Event (1)
- Windows Authentication (1)
- windows.event (1)
- WIP (1)
- WITH (2)
- With Parameter (1)
- Without Data (1)
- word-wrap: break-word; (1)
- www. (1)
- www2 (1)
- XML (1)
- XML cannot be the whole program (1)
- XML Tag (1)
- Year-To-Date (1)
- ZEROFILL (1)
- zh-Hans (1)
- zh-Hant (1)
- σ (1)