Both MySQL and PHP/Java support the Unicode, namely UTF-8. To be able to store into and retrieve these Unicode characters in MySQL database, one would need to do following things:
MySQL needs to be informed through PHP/Java. So, following code needs to be placed just after the connection. If the connection is centralized, this code is better to be in that centralized file:
PHP: mysql_query("SET NAMES 'UTF8'");
Java: stmt.execute("SET NAMES 'utf8'");
For all your PHP files generates HTML scripts, please include following code at top of the file:
header("Content-Type: text/html; charset=UTF-8");
For all your HTML files, following script is needed in the head of HTML:
‹ meta http-equiv="Content-Type" content="text/html; charset=UTF-8" ›
Done. Please note, in first piece of PHP code, it is UTF8 and in second PHP code and last HTML script it is UTF-8. Else, it won’t work properly.
If your codes include any of following piece, simply remove it:
PHP: mysql_query("SET CHARACTER SET 'UTF8'");
Java: stmt.execute("SET CHARACTER SET 'utf8'");
CSS and Link
CSS can be written two ways. The first way has selector and second way does not have selector but class selectors only.
First way:
sty
{ color: #333333;
}
First way covers every situation of class selectors. One for all. However, if a particular situation, say “hover” is needed to be excluded from the uniform of selector, it can be added as an overwrite:
sty
{ color: #333333;
}
sty:hover
{ color: #CCCCCC;
}
Second way:
sty:link
{ color: #333333;
}
sty:visited
{ color: #FFFFFF;
}
sty:hover
{ color: #CCCCCC;
}
sty:active
{ color: #333333;
}
Using second way, one must specify every situation. Else, it would inherited from the rule that is used by the element in which the link exists. For 2nd way, there is an order requirement: LoVe/HAte, else, it won't work.
The CSS can be not only used for link, but text as well. However, to enable class selectors work properly, the anchor must be exist:
‹a href="http://koncordpartners.blogspot.com" class="sty"›
This is the anchor started with letter “a”. If no link needed, use this href: href="#".
‹/a›
It also means applying class at table or cell/td level won'd work.
http://www.projectseven.com/tutorials/css/pseudoclasses/
First way:
sty
{ color: #333333;
}
First way covers every situation of class selectors. One for all. However, if a particular situation, say “hover” is needed to be excluded from the uniform of selector, it can be added as an overwrite:
sty
{ color: #333333;
}
sty:hover
{ color: #CCCCCC;
}
Second way:
sty:link
{ color: #333333;
}
sty:visited
{ color: #FFFFFF;
}
sty:hover
{ color: #CCCCCC;
}
sty:active
{ color: #333333;
}
Using second way, one must specify every situation. Else, it would inherited from the rule that is used by the element in which the link exists. For 2nd way, there is an order requirement: LoVe/HAte, else, it won't work.
The CSS can be not only used for link, but text as well. However, to enable class selectors work properly, the anchor must be exist:
‹a href="http://koncordpartners.blogspot.com" class="sty"›
This is the anchor started with letter “a”. If no link needed, use this href: href="#".
‹/a›
It also means applying class at table or cell/td level won'd work.
http://www.projectseven.com/tutorials/css/pseudoclasses/
Number of Elements of Array
Number of elements of array is very usual, which can be used as control for the loop or other purposes. Function to get it in JavaScript is varArray.length. In PHP it is count($ varArray) or sizeof($ varArray). Since sizeof() is just an alias of count(), it is suggested using count(), since sizeof() has other meaning in other languages.
The problem happens when array is undefined/not exist. In JavaScript .length would generate a fatal error. So, for save play, it is suggested always using follow codes:
var len = 0;
if ('undefined'!=typeof(varArray)) len = varArray.length;
In PHP count() would generate a return value of 0 together with a non-fatal error message, which would cause even more serious problem because of its non-fatal nature. Fortunately, PHP provides an easy non-fatal error message suppress method “@”:
@count($varArray);
It is very useful because it enables you to always associate @ with count(). And please do so.
For multidimensional array, the usage will be exactly same; and can be used for particular dimension, such as varArray[i] or $varArray[i].
The problem happens when array is undefined/not exist. In JavaScript .length would generate a fatal error. So, for save play, it is suggested always using follow codes:
var len = 0;
if ('undefined'!=typeof(varArray)) len = varArray.length;
In PHP count() would generate a return value of 0 together with a non-fatal error message, which would cause even more serious problem because of its non-fatal nature. Fortunately, PHP provides an easy non-fatal error message suppress method “@”:
@count($varArray);
It is very useful because it enables you to always associate @ with count(). And please do so.
For multidimensional array, the usage will be exactly same; and can be used for particular dimension, such as varArray[i] or $varArray[i].
Test Various “Nothings” in PHP
Following php codes is to test six different “nothing”variables together with popular functions, plus number 1 as control.
‹?php
$undefinedVar;
$nullVar = null;
$strEmpty = "";
$strChanged = "initial value";
$numZero = 0;
$numChanged = 1;
$numOne = 1;
$strChanged = null;
$numChanged = null;
echo "‹br/›11. undefinedVar: (".$undefinedVar.")";
echo "‹br/›12. nullVar: (".$nullVar.")";
echo "‹br/›13. strEmpty: (".$strEmpty.")";
echo "‹br/›14. strChanged: (".$strChanged.")";
echo "‹br/›15. numZero: (".$numZero.")";
echo "‹br/›16. numChanged: (".$numChanged.")";
echo "‹br/›21. is_null-undefinedVar: (".is_null($undefinedVar).")";
echo "‹br/›22. is_null-nullVar: (".is_null($nullVar).")";
echo "‹br/›23. is_null-strEmpty: (".is_null($strEmpty).")";
echo "‹br/›24. is_null-strChanged: (".is_null($strChanged).")";
echo "‹br/›25. is_null-numZero: (".is_null($numZero).")";
echo "‹br/›26. is_null-numChanged: (".is_null($numChanged).")";
echo "‹br/›31. isset-undefinedVar: (".isset($undefinedVar).")";
echo "‹br/›32. isset-nullVar: (".isset($nullVar).")";
echo "‹br/›33. isset-strEmpty: (".isset($strEmpty).")";
echo "‹br/›34. isset-strChanged: (".isset($strChanged).")";
echo "‹br/›35. isset-numZero: (".isset($numZero).")";
echo "‹br/›36. isset-numChanged: (".isset($numChanged).")";
echo "‹br/›47. TRUE: (".TRUE.")";
echo "‹br/›48. FALSE: (".FALSE.")";
echo "‹br/›51. empty-undefinedVar: (".empty($undefinedVar).")";
echo "‹br/›52. empty-nullVar: (".empty($nullVar).")";
echo "‹br/›53. empty-strEmpty: (".empty($strEmpty).")";
echo "‹br/›54. empty-strChanged: (".empty($strChanged).")";
echo "‹br/›55. empty-numZero: (".empty($numZero).")";
echo "‹br/›56. empty-numChanged: (".empty($numChanged).")";
echo "‹br/›57. empty-numOne: (".empty($numOne).")";
echo "‹br/›61. defined-undefinedVar: (".defined($undefinedVar).")";
echo "‹br/›62. defined-nullVar: (".defined($nullVar).")";
echo "‹br/›63. defined-strEmpty: (".defined($strEmpty).")";
echo "‹br/›64. defined-strChanged: (".defined($strChanged).")";
echo "‹br/›65. defined-numZero: (".defined($numZero).")";
echo "‹br/›66. defined-numChanged: (".defined($numChanged).")";
echo "‹br/›71. strlen-trim-undefinedVar: (".strlen(trim($undefinedVar)).")";
echo "‹br/›72. strlen-trim-nullVar: (".strlen(trim($nullVar)).")";
echo "‹br/›73. strlen-trim-strEmpty: (".strlen(trim($strEmpty)).")";
echo "‹br/›74. strlen-trim-strChanged: (".strlen(trim($strChanged)).")";
echo "‹br/›75. strlen-trim-numZero: (".strlen(trim($numZero)).")";
echo "‹br/›76. strlen-trim-numChanged: (".strlen(trim($numChanged)).")";
?›
unset() does not work for any one of them. Therefore, it had been excluded.
Test results in both Firefox and IE are identical, as follows:
11. undefinedVar: ()
12. nullVar: ()
13. strEmpty: ()
14. strChanged: ()
15. numZero: (0)
16. numChanged: ()
21. is_null-undefinedVar: (1)
22. is_null-nullVar: (1)
23. is_null-strEmpty: ()
24. is_null-strChanged: (1)
25. is_null-numZero: ()
26. is_null-numChanged: (1)
31. isset-undefinedVar: ()
32. isset-nullVar: ()
33. isset-strEmpty: (1)
34. isset-strChanged: ()
35. isset-numZero: (1)
36. isset-numChanged: ()
47. TRUE: (1)
48. FALSE: ()
51. empty-undefinedVar: (1)
52. empty-nullVar: (1)
53. empty-strEmpty: (1)
54. empty-strChanged: (1)
55. empty-numZero: (1)
56. empty-numChanged: (1)
57. empty-numOne: ()
61. defined-undefinedVar: ()
62. defined-nullVar: ()
63. defined-strEmpty: ()
64. defined-strChanged: ()
65. defined-numZero: ()
66. defined-numChanged: ()
71. strlen-trim-undefinedVar: (0)
72. strlen-trim-nullVar: (0)
73. strlen-trim-strEmpty: (0)
74. strlen-trim-strChanged: (0)
75. strlen-trim-numZero: (1)
76. strlen-trim-numChanged: (0)
Observed, included but not limited to this test:
1. Results in Firefox and IE are exactly same. However, if an null variable passed from JavaScript to PHP, in IE, it would be shown as a string "null". In a real case scenario, a variable is to pass from form feeding in HTML/JavaScript to PHP. However, it had only be assigned with value “null”. It supposes an integer data type. Unfortunately, in is_null() in PHP, it results not null, while echo shows “null” in IE and nothing in Firefox.
2. unset() does not work at all for all of them.
3. is_null() works fine. It however regards empty string as not null, which should be.
4. isset() works fine. It regards empty string as set.
5. is_null() is just opposite to isset().
6. empty() works for all, including regarding number 0 as empty.
7. defined() is to detect if a constant string exists, which does works fine here, since no constant string here.
8. strlen(trim()) does work fine except number 0, which should be.
Conclusion:
First of all, never use null in JavaScript where it supposed to be a string. Use '' instead. This is because in IE, when JavaScript passes string to PHP, null would become 'null'.
1. If one want to include everything, null, empty string, number 0, and even string “0”, the best approach is to use if(empty($var)).
2. If one wants to include null and empty string and exclude number 0, if(0==strlen(trim($var)) might be best approach. However, this approach is unable to detect the string "null".
3. If one wants to include null and number 0 and exclude empty string, the best approach maybe if(is_null($var) || 0==$var).
4. If one wants to only include null and exclude empty string and number 0, the best approach is if(is_null($var)). However, since both JavaScript and PHP does not tell the data type when declare a variable, it somehow easily to be mixed up the undefined variable or null variable with empty string. It is therefore suggested extra caution shall be applied to exclude empty string.
5. In most case people dealing with null would include undefined variable, null variable, empty string in JavaScript and PHP. For special case mentioned above for IE, it would includes string "null" as well, which isn't covered by Conclustion 2. So, the possible most save approach is put two together:
if(0==strlen(trim($var)) || "null"==$var)
6. To deal with undefined array, please refer to http://koncordpartners.blogspot.com/2009/12/number-of-elements-of-array.html.
http://ca2.php.net/manual/en/function.empty.php
‹?php
$undefinedVar;
$nullVar = null;
$strEmpty = "";
$strChanged = "initial value";
$numZero = 0;
$numChanged = 1;
$numOne = 1;
$strChanged = null;
$numChanged = null;
echo "‹br/›11. undefinedVar: (".$undefinedVar.")";
echo "‹br/›12. nullVar: (".$nullVar.")";
echo "‹br/›13. strEmpty: (".$strEmpty.")";
echo "‹br/›14. strChanged: (".$strChanged.")";
echo "‹br/›15. numZero: (".$numZero.")";
echo "‹br/›16. numChanged: (".$numChanged.")";
echo "‹br/›21. is_null-undefinedVar: (".is_null($undefinedVar).")";
echo "‹br/›22. is_null-nullVar: (".is_null($nullVar).")";
echo "‹br/›23. is_null-strEmpty: (".is_null($strEmpty).")";
echo "‹br/›24. is_null-strChanged: (".is_null($strChanged).")";
echo "‹br/›25. is_null-numZero: (".is_null($numZero).")";
echo "‹br/›26. is_null-numChanged: (".is_null($numChanged).")";
echo "‹br/›31. isset-undefinedVar: (".isset($undefinedVar).")";
echo "‹br/›32. isset-nullVar: (".isset($nullVar).")";
echo "‹br/›33. isset-strEmpty: (".isset($strEmpty).")";
echo "‹br/›34. isset-strChanged: (".isset($strChanged).")";
echo "‹br/›35. isset-numZero: (".isset($numZero).")";
echo "‹br/›36. isset-numChanged: (".isset($numChanged).")";
echo "‹br/›47. TRUE: (".TRUE.")";
echo "‹br/›48. FALSE: (".FALSE.")";
echo "‹br/›51. empty-undefinedVar: (".empty($undefinedVar).")";
echo "‹br/›52. empty-nullVar: (".empty($nullVar).")";
echo "‹br/›53. empty-strEmpty: (".empty($strEmpty).")";
echo "‹br/›54. empty-strChanged: (".empty($strChanged).")";
echo "‹br/›55. empty-numZero: (".empty($numZero).")";
echo "‹br/›56. empty-numChanged: (".empty($numChanged).")";
echo "‹br/›57. empty-numOne: (".empty($numOne).")";
echo "‹br/›61. defined-undefinedVar: (".defined($undefinedVar).")";
echo "‹br/›62. defined-nullVar: (".defined($nullVar).")";
echo "‹br/›63. defined-strEmpty: (".defined($strEmpty).")";
echo "‹br/›64. defined-strChanged: (".defined($strChanged).")";
echo "‹br/›65. defined-numZero: (".defined($numZero).")";
echo "‹br/›66. defined-numChanged: (".defined($numChanged).")";
echo "‹br/›71. strlen-trim-undefinedVar: (".strlen(trim($undefinedVar)).")";
echo "‹br/›72. strlen-trim-nullVar: (".strlen(trim($nullVar)).")";
echo "‹br/›73. strlen-trim-strEmpty: (".strlen(trim($strEmpty)).")";
echo "‹br/›74. strlen-trim-strChanged: (".strlen(trim($strChanged)).")";
echo "‹br/›75. strlen-trim-numZero: (".strlen(trim($numZero)).")";
echo "‹br/›76. strlen-trim-numChanged: (".strlen(trim($numChanged)).")";
?›
unset() does not work for any one of them. Therefore, it had been excluded.
Test results in both Firefox and IE are identical, as follows:
11. undefinedVar: ()
12. nullVar: ()
13. strEmpty: ()
14. strChanged: ()
15. numZero: (0)
16. numChanged: ()
21. is_null-undefinedVar: (1)
22. is_null-nullVar: (1)
23. is_null-strEmpty: ()
24. is_null-strChanged: (1)
25. is_null-numZero: ()
26. is_null-numChanged: (1)
31. isset-undefinedVar: ()
32. isset-nullVar: ()
33. isset-strEmpty: (1)
34. isset-strChanged: ()
35. isset-numZero: (1)
36. isset-numChanged: ()
47. TRUE: (1)
48. FALSE: ()
51. empty-undefinedVar: (1)
52. empty-nullVar: (1)
53. empty-strEmpty: (1)
54. empty-strChanged: (1)
55. empty-numZero: (1)
56. empty-numChanged: (1)
57. empty-numOne: ()
61. defined-undefinedVar: ()
62. defined-nullVar: ()
63. defined-strEmpty: ()
64. defined-strChanged: ()
65. defined-numZero: ()
66. defined-numChanged: ()
71. strlen-trim-undefinedVar: (0)
72. strlen-trim-nullVar: (0)
73. strlen-trim-strEmpty: (0)
74. strlen-trim-strChanged: (0)
75. strlen-trim-numZero: (1)
76. strlen-trim-numChanged: (0)
Observed, included but not limited to this test:
1. Results in Firefox and IE are exactly same. However, if an null variable passed from JavaScript to PHP, in IE, it would be shown as a string "null". In a real case scenario, a variable is to pass from form feeding in HTML/JavaScript to PHP. However, it had only be assigned with value “null”. It supposes an integer data type. Unfortunately, in is_null() in PHP, it results not null, while echo shows “null” in IE and nothing in Firefox.
2. unset() does not work at all for all of them.
3. is_null() works fine. It however regards empty string as not null, which should be.
4. isset() works fine. It regards empty string as set.
5. is_null() is just opposite to isset().
6. empty() works for all, including regarding number 0 as empty.
7. defined() is to detect if a constant string exists, which does works fine here, since no constant string here.
8. strlen(trim()) does work fine except number 0, which should be.
Conclusion:
First of all, never use null in JavaScript where it supposed to be a string. Use '' instead. This is because in IE, when JavaScript passes string to PHP, null would become 'null'.
1. If one want to include everything, null, empty string, number 0, and even string “0”, the best approach is to use if(empty($var)).
2. If one wants to include null and empty string and exclude number 0, if(0==strlen(trim($var)) might be best approach. However, this approach is unable to detect the string "null".
3. If one wants to include null and number 0 and exclude empty string, the best approach maybe if(is_null($var) || 0==$var).
4. If one wants to only include null and exclude empty string and number 0, the best approach is if(is_null($var)). However, since both JavaScript and PHP does not tell the data type when declare a variable, it somehow easily to be mixed up the undefined variable or null variable with empty string. It is therefore suggested extra caution shall be applied to exclude empty string.
5. In most case people dealing with null would include undefined variable, null variable, empty string in JavaScript and PHP. For special case mentioned above for IE, it would includes string "null" as well, which isn't covered by Conclustion 2. So, the possible most save approach is put two together:
if(0==strlen(trim($var)) || "null"==$var)
6. To deal with undefined array, please refer to http://koncordpartners.blogspot.com/2009/12/number-of-elements-of-array.html.
http://ca2.php.net/manual/en/function.empty.php
Are “Title” and "Top" Keywords in JavaScript?
No, actually they is not. However, please do not use them as name or id in HTML. Following problem has been notices:
‹input id=”title” type=”text” value”Title is forbidden” ›
When try to get value by document.getElementById('title').value, it generate null in IE. If one exchanges the “title” with other word, it works fine.
In Chrome, one case use top() as function name, it does not work while it works fine with IE and Firefox.
Very special!
http://aptana.com/reference/html/api/JSKeywords.index.html
‹input id=”title” type=”text” value”Title is forbidden” ›
When try to get value by document.getElementById('title').value, it generate null in IE. If one exchanges the “title” with other word, it works fine.
In Chrome, one case use top() as function name, it does not work while it works fine with IE and Firefox.
Very special!
http://aptana.com/reference/html/api/JSKeywords.index.html
Days in the Month in T-SQL
Number of days in the month are same as the last day’s date number in the month. Unlike PL/SQL, in T-SQL there is no function called last_day(). Following are two methods to get it:
Method 1, if you know the date:
DECLARE @Date datetime
SET @Date = '2009/12/10'
SELECT DAY(DATEADD(d,-1,dateadd(m,1,@Date))) AS 'Last day of the month'
Method 2, if you know the year and month number:
SELECT DAY(DATEADD(D, -1, DATEADD(M, 1, CONVERT(DATETIME, (CONVERT(VARCHAR, Yer) + '-' + CONVERT(VARCHAR, Mth) + '-01'))))) AS 'Last day of the month'
http://www.bigresource.com/MS_SQL-last_day-function-s5q435mI.html
Method 1, if you know the date:
DECLARE @Date datetime
SET @Date = '2009/12/10'
SELECT DAY(DATEADD(d,-1,dateadd(m,1,@Date))) AS 'Last day of the month'
Method 2, if you know the year and month number:
SELECT DAY(DATEADD(D, -1, DATEADD(M, 1, CONVERT(DATETIME, (CONVERT(VARCHAR, Yer) + '-' + CONVERT(VARCHAR, Mth) + '-01'))))) AS 'Last day of the month'
http://www.bigresource.com/MS_SQL-last_day-function-s5q435mI.html
Contain Data Layout in SQL Part for Reporting Services
Business Intelligence in both ORACLE and SQL Server has offered standard tools and settings, such as cube and dimensions. However, for complicated reports, these tools may not be very useful. The orthodox approach to this issue is to have a customized OLAP database built. In some case, even with OLAP database, complicated queries still needed. This issue does also cause the further problem of data layout in report. Here is an approach to deal with these issues. Basically, this approach does contain the task of data layout in SQL part to avoid complex programming in Stored Procedures.
Let us say a report is required to present a daily summary of patient movement within a State, with certain criteria. It requires 5 columns: Date, Sending Facility, Receiving Facility, total transports between these two facilities in that day [Transports], and the Daily Subtotal for whole State. Indeed, Daily Subtotal and Transports can share same column. However, for better visual effect, it is required to have separate columns. GROUP BY and other analytic functions can be used to generate numbers for Transports and Daily Subtotal. However, for the sake of data layout in report, it is suggested only GROUP BY is used. As at today, almost all other analytic functions just generate undesired data layout, for instance, extra but unneeded columns from PIVOT.
The data was not located in a single table, so joins are necessary. Since the selection criteria (WHERE Conditions) are not strictly have one-to-one relationship, the joins therefore are Outer Joins to ensure needed records won’t be excluded. Here is FROM clause:
FROM dbo.transfer t
LEFT JOIN dbo.healthcare_facility s ON s.healthcare_facility_id = t.sending_facility_id
LEFT JOIN dbo.healthcare_facility r ON r.healthcare_facility_id = t.receiving_facility_id
LEFT JOIN ……
The sending facility and receiving facility indeed is in same table. So, it had been outer joined twice with flag as s and r.
Because of one-to-more relationship, it is for sure multiple rows will be generated for some records. So, mechanism to distinct records is needed. Unfortunately, DISTINCT function does not work with GROUP BY. So, an inner query is used strictly only selecting the essential fields together with DISTINCT:
( SELECT DISTINCT DATEADD(HH, 12, DATEDIFF(DD, 0, t.add_datetime)) Entry_Date
, m.mt_number [MT#]
, s.healthcare_facility_name Sending_Facility
, r.healthcare_facility_name Receiving_Facility
FROM dbo.transfer t
LEFT JOIN dbo.healthcare_facility s ON s.healthcare_facility_id = t.sending_facility_id
LEFT JOIN dbo.healthcare_facility r ON r.healthcare_facility_id = t.receiving_facility_id
LEFT JOIN …
WHERE …
) AS Distinct_Q
Do not forget to add “AS [name_of_inner_query]” at the end of inner query, which is required by Transact-SQL. DATEDIFF(DD, 0, t.add_datetime) is used here to uniform the time during the date enabling the use of GROUP BY. DATEADD(HH, 12, …) will be explained late. If Distinct_Q contains less fields needed by next step, it is neccessary to join back to retrieve the missing fields.
Next step is to use GROUP BY:
( SELECT Entry_Date
, Sending_Facility
, Receiving_Facility
, COUNT(*) Daily_Transports
FROM
(…) AS Distinct_Q
GROUP BY Entry_Date
, Sending_Facility
, Receiving_Facility
) AS Detail_Q
So far, we generate all essential parts of the report except the Daily Subtotal. We can use same structure for Daily Subtotal. Since inner query Distinct_Q used twice, it is worthwhile to move this temporary resultset to the head with WITH:
WITH Distinct_Q
AS
(…
)
(SELECT DATEADD(HH, -1, Entry_Date) E_Date
, COUNT(*) Daily_Subtotal
FROM Distinct_Q
GROUP BY Entry_Date
) AS Daily_Subtotal_Q
Note, DATEADD(HH, -1, Entry_Date) is used again here.
We can use UNION to put two temporary resultsets Detail_Q and Daily_Subtotal_Q together:
SELECT Entry_Date [Entry Date]
, Transfer_Status [Transfer Status]
, Sending_Facility [Sending Facility]
, Receiving_Facility [Receiving Facility]
, CAST(Daily_Transports AS VARCHAR(3)) Transports
, '' [Daily Subtotal]
FROM
(…) AS Detail_Q
UNION
SELECT E_Date [Entry Date]
, '' [Transfer Status]
, 'Daily Subtotal' [Sending Facility]
, '' [Receiving Facility]
, '' Transports
, CAST(Daily_Subtotal AS VARCHAR(3)) [Daily Subtotal]
FROM
(…) AS Daily_Subtotal_Q
The purpose of CAST(… AS VARCHAR(3)) used here is to avoid the empty string '' automatically turns to 0 after UNION. Field [Daily Subtotal] in Detail_Q is string data type and Daily_Subtotal_Q is number. So does for field [Transports].
The final step to to add ORDER BY at the end:
ORDER BY [Entry Date] DESC
, [Transfer Status]
, [Sending Facility]
, [Receiving Facility]
, [Transports]
, [Daily Subtotal]
GO
It is time to explain why using DATEADD() function above. Since [Entry Date] is to be sorted DESCENT-ly, one hour difference as result of two DATEADD() functions could place row of ‘Daily Subtotal’ at the end of every rows for that day.
Here is completed SQL script:
WITH Distinct_Q
AS
( SELECT DISTINCT DATEADD(HH, 12, DATEDIFF(DD, 0, t.add_datetime)) Entry_Date
, m.mt_number [MT#]
, s.healthcare_facility_name Sending_Facility
, r.healthcare_facility_name Receiving_Facility
FROM dbo.transfer t
LEFT JOIN dbo.healthcare_facility s ON s.healthcare_facility_id = t.sending_facility_id
LEFT JOIN dbo.healthcare_facility r ON r.healthcare_facility_id = t.receiving_facility_id
LEFT JOIN …
WHERE …
)
SELECT Entry_Date [Entry Date]
, Transfer_Status [Transfer Status]
, Sending_Facility [Sending Facility]
, Receiving_Facility [Receiving Facility]
, CAST(Daily_Transports AS VARCHAR(3)) Transports
, '' [Daily Subtotal]
FROM
( SELECT Entry_Date
, Sending_Facility
, Receiving_Facility
, COUNT(*) Daily_Transports
FROM Distinct_Q
GROUP BY Entry_Date
, Sending_Facility
, Receiving_Facility
) AS Detail_Q
UNION
SELECT E_Date [Entry Date]
, '' [Transfer Status]
, 'Daily Subtotal' [Sending Facility]
, '' [Receiving Facility]
, '' Transports
, CAST(Daily_Subtotal AS VARCHAR(3)) [Daily Subtotal]
FROM
(SELECT DATEADD(HH, -1, Entry_Date) E_Date
, COUNT(*) Daily_Subtotal
FROM Distinct_Q
GROUP BY Entry_Date
) AS Daily_Subtotal_Q
ORDER BY [Entry Date] DESC
, [Transfer Status]
, [Sending Facility]
, [Receiving Facility]
, [Transports]
, [Daily Subtotal]
GO
This blog is to illustrate how to manipulate the data layout in report simply by use SQL. You can also refer to http://koncordpartners.blogspot.com/2009/08/practical-case-of-transpose-query.html.
http://koncordpartners.blogspot.com/2010/12/special-function-of-order-by-clause-in.html
Let us say a report is required to present a daily summary of patient movement within a State, with certain criteria. It requires 5 columns: Date, Sending Facility, Receiving Facility, total transports between these two facilities in that day [Transports], and the Daily Subtotal for whole State. Indeed, Daily Subtotal and Transports can share same column. However, for better visual effect, it is required to have separate columns. GROUP BY and other analytic functions can be used to generate numbers for Transports and Daily Subtotal. However, for the sake of data layout in report, it is suggested only GROUP BY is used. As at today, almost all other analytic functions just generate undesired data layout, for instance, extra but unneeded columns from PIVOT.
The data was not located in a single table, so joins are necessary. Since the selection criteria (WHERE Conditions) are not strictly have one-to-one relationship, the joins therefore are Outer Joins to ensure needed records won’t be excluded. Here is FROM clause:
FROM dbo.transfer t
LEFT JOIN dbo.healthcare_facility s ON s.healthcare_facility_id = t.sending_facility_id
LEFT JOIN dbo.healthcare_facility r ON r.healthcare_facility_id = t.receiving_facility_id
LEFT JOIN ……
The sending facility and receiving facility indeed is in same table. So, it had been outer joined twice with flag as s and r.
Because of one-to-more relationship, it is for sure multiple rows will be generated for some records. So, mechanism to distinct records is needed. Unfortunately, DISTINCT function does not work with GROUP BY. So, an inner query is used strictly only selecting the essential fields together with DISTINCT:
( SELECT DISTINCT DATEADD(HH, 12, DATEDIFF(DD, 0, t.add_datetime)) Entry_Date
, m.mt_number [MT#]
, s.healthcare_facility_name Sending_Facility
, r.healthcare_facility_name Receiving_Facility
FROM dbo.transfer t
LEFT JOIN dbo.healthcare_facility s ON s.healthcare_facility_id = t.sending_facility_id
LEFT JOIN dbo.healthcare_facility r ON r.healthcare_facility_id = t.receiving_facility_id
LEFT JOIN …
WHERE …
) AS Distinct_Q
Do not forget to add “AS [name_of_inner_query]” at the end of inner query, which is required by Transact-SQL. DATEDIFF(DD, 0, t.add_datetime) is used here to uniform the time during the date enabling the use of GROUP BY. DATEADD(HH, 12, …) will be explained late. If Distinct_Q contains less fields needed by next step, it is neccessary to join back to retrieve the missing fields.
Next step is to use GROUP BY:
( SELECT Entry_Date
, Sending_Facility
, Receiving_Facility
, COUNT(*) Daily_Transports
FROM
(…) AS Distinct_Q
GROUP BY Entry_Date
, Sending_Facility
, Receiving_Facility
) AS Detail_Q
So far, we generate all essential parts of the report except the Daily Subtotal. We can use same structure for Daily Subtotal. Since inner query Distinct_Q used twice, it is worthwhile to move this temporary resultset to the head with WITH:
WITH Distinct_Q
AS
(…
)
(SELECT DATEADD(HH, -1, Entry_Date) E_Date
, COUNT(*) Daily_Subtotal
FROM Distinct_Q
GROUP BY Entry_Date
) AS Daily_Subtotal_Q
Note, DATEADD(HH, -1, Entry_Date) is used again here.
We can use UNION to put two temporary resultsets Detail_Q and Daily_Subtotal_Q together:
SELECT Entry_Date [Entry Date]
, Transfer_Status [Transfer Status]
, Sending_Facility [Sending Facility]
, Receiving_Facility [Receiving Facility]
, CAST(Daily_Transports AS VARCHAR(3)) Transports
, '' [Daily Subtotal]
FROM
(…) AS Detail_Q
UNION
SELECT E_Date [Entry Date]
, '' [Transfer Status]
, 'Daily Subtotal' [Sending Facility]
, '' [Receiving Facility]
, '' Transports
, CAST(Daily_Subtotal AS VARCHAR(3)) [Daily Subtotal]
FROM
(…) AS Daily_Subtotal_Q
The purpose of CAST(… AS VARCHAR(3)) used here is to avoid the empty string '' automatically turns to 0 after UNION. Field [Daily Subtotal] in Detail_Q is string data type and Daily_Subtotal_Q is number. So does for field [Transports].
The final step to to add ORDER BY at the end:
ORDER BY [Entry Date] DESC
, [Transfer Status]
, [Sending Facility]
, [Receiving Facility]
, [Transports]
, [Daily Subtotal]
GO
It is time to explain why using DATEADD() function above. Since [Entry Date] is to be sorted DESCENT-ly, one hour difference as result of two DATEADD() functions could place row of ‘Daily Subtotal’ at the end of every rows for that day.
Here is completed SQL script:
WITH Distinct_Q
AS
( SELECT DISTINCT DATEADD(HH, 12, DATEDIFF(DD, 0, t.add_datetime)) Entry_Date
, m.mt_number [MT#]
, s.healthcare_facility_name Sending_Facility
, r.healthcare_facility_name Receiving_Facility
FROM dbo.transfer t
LEFT JOIN dbo.healthcare_facility s ON s.healthcare_facility_id = t.sending_facility_id
LEFT JOIN dbo.healthcare_facility r ON r.healthcare_facility_id = t.receiving_facility_id
LEFT JOIN …
WHERE …
)
SELECT Entry_Date [Entry Date]
, Transfer_Status [Transfer Status]
, Sending_Facility [Sending Facility]
, Receiving_Facility [Receiving Facility]
, CAST(Daily_Transports AS VARCHAR(3)) Transports
, '' [Daily Subtotal]
FROM
( SELECT Entry_Date
, Sending_Facility
, Receiving_Facility
, COUNT(*) Daily_Transports
FROM Distinct_Q
GROUP BY Entry_Date
, Sending_Facility
, Receiving_Facility
) AS Detail_Q
UNION
SELECT E_Date [Entry Date]
, '' [Transfer Status]
, 'Daily Subtotal' [Sending Facility]
, '' [Receiving Facility]
, '' Transports
, CAST(Daily_Subtotal AS VARCHAR(3)) [Daily Subtotal]
FROM
(SELECT DATEADD(HH, -1, Entry_Date) E_Date
, COUNT(*) Daily_Subtotal
FROM Distinct_Q
GROUP BY Entry_Date
) AS Daily_Subtotal_Q
ORDER BY [Entry Date] DESC
, [Transfer Status]
, [Sending Facility]
, [Receiving Facility]
, [Transports]
, [Daily Subtotal]
GO
This blog is to illustrate how to manipulate the data layout in report simply by use SQL. You can also refer to http://koncordpartners.blogspot.com/2009/08/practical-case-of-transpose-query.html.
http://koncordpartners.blogspot.com/2010/12/special-function-of-order-by-clause-in.html
Structure in Web Development (1)
This is study notes of web development involving the relationship and value passing between technologies.
HTML, Hyper Text Markup Language, as its name suggested is a markup language, which does formatting for the document; such as font, border format for cells in table, etc. There are two parts in HTML, head section and body section. Head contains all metadata, controls and the body is the document to be presented in web browser. When URL called by browser, whole HTML file is downloaded in the browser’ computer, which means it is a client side process.
To be easily and programmatically changed or updated, all formats can be centrally managed by a separate file, with extension of .css. This is the file of Cascading Style Sheets, or CSS. The inner link between two files is done through the following declaration to be included in the head of HTML:
‹link rel="stylesheet" type="text/css" href="cssFilename.css"›
In addition, in each division of HTML, “class=”classInCSS”” is needed to point the format class in CSS file. There is no value passing between two files.
Both HTML and CSS do not have programming functionality. To add the programming functionality, JavaScript can be added in HTML. Since programming functions are not of the presentation, it therefore locates in the head of HTML. However, when JavaScript consists too much contents, it can be separated as a file with extension of .js. The inner link between two files is done through the following declaration to be included in the head of HTML:
‹script type='text/javascript' language='Javascript' src='javascriptFileName.js' ›‹/script›
When JavaScript file is called by the HTML in browser’s computer, the whole file is downloaded too. So, JavaScript is also the client side process. Since contents of HTML may need to be changed frequently, separation of HTML and JavaScript may help to improve the refresh speed because programming functions may not change so frequently. This is another reason why JavaScript needs to be an independent file. However, this reason may not sustainable in DHTML scenario, to be mentioned late. At this stage, we deal with the HTML with static contents.
Functions in JavaScript need to be invoked. It can be done through window events, such as mouse move, mouse over, mouse out, mouse click or HTML page on load, etc; or through the calling from another funcation. Since HTML content is static, when value in HTML need to be passed to JavaScript, it is no need to be dealt specifically. Programmer can just use that value when write JavaScript file. In other words, there is no value passed from HTML to JavaScript, apart from invoking action. However, if value passing from JavaScript to HTML is needed, it can be done through “documnt.write()” and “.innerHTML =” methods. “documnt.write()” is located within the body of HTML. Since HTML body is for presentation purpose, to use “documnt.write()” one much first declare it is of JavaScript scripts:
‹script type="text/javascript"›
document.write(' javascriptVariable ');
‹/script›
The major difference between “documnt.write()” and “.innerHTML =” is “documnt.write()” does not need trigger to be active, simply because it is located in the body of HTML. When HTML page loaded, it is invoked. On contrast, “.innerHTML =” is located in JavaScript section/file, it needs to be called either by window events or other functions. Generally, “.innerHTML =” method is recommended. For instance:
document.getElementById('aDivision').innerHTML = javascriptVariable;
By this way, the value javascriptVariable generated from JavaScript functions is delivered to a HTML division called “aDivision”. Since HTML is only the markup language, there will be no variable involved in pure HTML. Variables need to be declared in JavaScript. Indeed, by this way, HTML can have variables. In addition, by this way, the delivered can be not only a value of single variable, but also more complicated contents:
var dynamicTable = ‘‹table›‹tr›‹td›’ + javascriptVariable + ‘‹/td›‹/tr›‹/table›’;
document.getElementById('aDivision').innerHTML = dynamicTable;
Please note, variable dynamicTable now contains some HTML codes, as string. The actual delivered now is no longer the single value but a complete table. This is very much same concept of Dynamic SQL if one is familiar with the database. This indeed is the basic concept of Dynamic HTML, or DHTML, for which the contents together with associated HTML codes are dynamically generated by the running of JavaScript functions.
Each HTML together with its associated CSS and JavaScript files are an independent program. If there are more than one HTML pages in a website, how they are structured? Since HTML and CSS files do not really involve the value passing, Multiple HTML pages can share a single CSS file. One HTML can have more than one JavaScript files by declare both of them in the head:
‹script type='text/javascript' language='Javascript' src='javascriptFile_1.js' ›‹/script›
‹script type='text/javascript' language='Javascript' src='javascriptFile_2.js' ›‹/script›
When a function is called, the browser will first search if “this” file have this function, if not, it would automatically goes to other JavaScript files declared in same HTML file.
It is not recommended to share JavaScript file between HTML pages. Because invoking any function is through window events, such as on page load etc. Sharing JavaScript file just confuses the computer and yourself, on which page load, for instance. Window events such as “onload” is very much HTML page specified and is not generic. However, for those generic functions with absolutely no window events involved, it can be aggregated into a JavaScript file to be shared by multiple HTML files.
Since each HTML page is an independent program, access to other’s functions or passing value between HTML pages is not recommended, for the sake of programming convention as well as security concern. In special cases like one HTML page (child) was opened by another HTML page (parent), it can be done with limitation. For instance:
In parent JavaScript file:
var windowChild = window.open(url); // “url” is the string variable.
windowChild.close();
In this example, parent JavaScript opens a new HTML window and close it immediately.
In child JavaScript file:
var valueFromParent = window.opener.valuePassToChild;
Child HTML can thus access the value of a variable in parent HTML. In case the child window is opened within the iFrame of parent, it is :
var valueFromParent = parent.valuePassToChild;
Please take special note, this syntax is also true for object reference passing through HTML pages. We know that the reference acts as pointer to point to original variable rather than make a copy, any using of the reference, valueFromParent here, is indeed referencing back to parent HTML ‘s object. It does generate the issue of speed. For details, see http://koncordpartners.blogspot.com/. So, please do apply this to the object other than simple variable, such as array.
Following is the method when parent window calls functions located in iFrame child:
document.getElementById('iFrameID').contentWindow
.functionInIFrame(parameter);
If one include this into another function, that function indeed can be called by same or other iFrame windows:
In parent JavaScript file:
function crossWindow(parameter)
{ document.getElementById('iFrameName').contentWindow
.functionInIFrame(parameter);
};
In iFrame file:
crossWindow(parameter);
Unfortunate, the string iFrameName should not be variable, otherwise dynamic function will be involved. Furthermore, dynamic reference passing does never work properly. It therefore dynamic reference and dynamic function should be avoid. In this example, one can use “if” statement to pick up right inner function:
function crossWindow(option, parameter)
{ if (1==option) document.getElementById('iFrameName_1').contentWindow
.functionInIFrame(parameter)
else document.getElementById('iFrameName_2').contentWindow
.functionInIFrame(parameter);
};
When “parameter” is reference to variable located parent window, things become little complex. JavaScript indeed regards it is about the variable in iFrame window. One needs to declare parameter locally first:
var parameter = parent.paraInParent;
What about in iFrame child window, calling corssWindow() function is through dynamic insertion? Something like:
window.onload = function()
{ document.getElementById('dynamicContent').innerHTML = '‹a href="#" onclick="parent.crossWindow(‘ + parameter + ‘)"›Click here to run function in parent window‹/a›';
};
No, it would never work properly if parameter is the reference referring back to another variable in parent window.
(To be continued)
HTML, Hyper Text Markup Language, as its name suggested is a markup language, which does formatting for the document; such as font, border format for cells in table, etc. There are two parts in HTML, head section and body section. Head contains all metadata, controls and the body is the document to be presented in web browser. When URL called by browser, whole HTML file is downloaded in the browser’ computer, which means it is a client side process.
To be easily and programmatically changed or updated, all formats can be centrally managed by a separate file, with extension of .css. This is the file of Cascading Style Sheets, or CSS. The inner link between two files is done through the following declaration to be included in the head of HTML:
‹link rel="stylesheet" type="text/css" href="cssFilename.css"›
In addition, in each division of HTML, “class=”classInCSS”” is needed to point the format class in CSS file. There is no value passing between two files.
Both HTML and CSS do not have programming functionality. To add the programming functionality, JavaScript can be added in HTML. Since programming functions are not of the presentation, it therefore locates in the head of HTML. However, when JavaScript consists too much contents, it can be separated as a file with extension of .js. The inner link between two files is done through the following declaration to be included in the head of HTML:
‹script type='text/javascript' language='Javascript' src='javascriptFileName.js' ›‹/script›
When JavaScript file is called by the HTML in browser’s computer, the whole file is downloaded too. So, JavaScript is also the client side process. Since contents of HTML may need to be changed frequently, separation of HTML and JavaScript may help to improve the refresh speed because programming functions may not change so frequently. This is another reason why JavaScript needs to be an independent file. However, this reason may not sustainable in DHTML scenario, to be mentioned late. At this stage, we deal with the HTML with static contents.
Functions in JavaScript need to be invoked. It can be done through window events, such as mouse move, mouse over, mouse out, mouse click or HTML page on load, etc; or through the calling from another funcation. Since HTML content is static, when value in HTML need to be passed to JavaScript, it is no need to be dealt specifically. Programmer can just use that value when write JavaScript file. In other words, there is no value passed from HTML to JavaScript, apart from invoking action. However, if value passing from JavaScript to HTML is needed, it can be done through “documnt.write()” and “.innerHTML =” methods. “documnt.write()” is located within the body of HTML. Since HTML body is for presentation purpose, to use “documnt.write()” one much first declare it is of JavaScript scripts:
‹script type="text/javascript"›
document.write(' javascriptVariable ');
‹/script›
The major difference between “documnt.write()” and “.innerHTML =” is “documnt.write()” does not need trigger to be active, simply because it is located in the body of HTML. When HTML page loaded, it is invoked. On contrast, “.innerHTML =” is located in JavaScript section/file, it needs to be called either by window events or other functions. Generally, “.innerHTML =” method is recommended. For instance:
document.getElementById('aDivision').innerHTML = javascriptVariable;
By this way, the value javascriptVariable generated from JavaScript functions is delivered to a HTML division called “aDivision”. Since HTML is only the markup language, there will be no variable involved in pure HTML. Variables need to be declared in JavaScript. Indeed, by this way, HTML can have variables. In addition, by this way, the delivered can be not only a value of single variable, but also more complicated contents:
var dynamicTable = ‘‹table›‹tr›‹td›’ + javascriptVariable + ‘‹/td›‹/tr›‹/table›’;
document.getElementById('aDivision').innerHTML = dynamicTable;
Please note, variable dynamicTable now contains some HTML codes, as string. The actual delivered now is no longer the single value but a complete table. This is very much same concept of Dynamic SQL if one is familiar with the database. This indeed is the basic concept of Dynamic HTML, or DHTML, for which the contents together with associated HTML codes are dynamically generated by the running of JavaScript functions.
Each HTML together with its associated CSS and JavaScript files are an independent program. If there are more than one HTML pages in a website, how they are structured? Since HTML and CSS files do not really involve the value passing, Multiple HTML pages can share a single CSS file. One HTML can have more than one JavaScript files by declare both of them in the head:
‹script type='text/javascript' language='Javascript' src='javascriptFile_1.js' ›‹/script›
‹script type='text/javascript' language='Javascript' src='javascriptFile_2.js' ›‹/script›
When a function is called, the browser will first search if “this” file have this function, if not, it would automatically goes to other JavaScript files declared in same HTML file.
It is not recommended to share JavaScript file between HTML pages. Because invoking any function is through window events, such as on page load etc. Sharing JavaScript file just confuses the computer and yourself, on which page load, for instance. Window events such as “onload” is very much HTML page specified and is not generic. However, for those generic functions with absolutely no window events involved, it can be aggregated into a JavaScript file to be shared by multiple HTML files.
Since each HTML page is an independent program, access to other’s functions or passing value between HTML pages is not recommended, for the sake of programming convention as well as security concern. In special cases like one HTML page (child) was opened by another HTML page (parent), it can be done with limitation. For instance:
In parent JavaScript file:
var windowChild = window.open(url); // “url” is the string variable.
windowChild.close();
In this example, parent JavaScript opens a new HTML window and close it immediately.
In child JavaScript file:
var valueFromParent = window.opener.valuePassToChild;
Child HTML can thus access the value of a variable in parent HTML. In case the child window is opened within the iFrame of parent, it is :
var valueFromParent = parent.valuePassToChild;
Please take special note, this syntax is also true for object reference passing through HTML pages. We know that the reference acts as pointer to point to original variable rather than make a copy, any using of the reference, valueFromParent here, is indeed referencing back to parent HTML ‘s object. It does generate the issue of speed. For details, see http://koncordpartners.blogspot.com/. So, please do apply this to the object other than simple variable, such as array.
Following is the method when parent window calls functions located in iFrame child:
document.getElementById('iFrameID').contentWindow
.functionInIFrame(parameter);
If one include this into another function, that function indeed can be called by same or other iFrame windows:
In parent JavaScript file:
function crossWindow(parameter)
{ document.getElementById('iFrameName').contentWindow
.functionInIFrame(parameter);
};
In iFrame file:
crossWindow(parameter);
Unfortunate, the string iFrameName should not be variable, otherwise dynamic function will be involved. Furthermore, dynamic reference passing does never work properly. It therefore dynamic reference and dynamic function should be avoid. In this example, one can use “if” statement to pick up right inner function:
function crossWindow(option, parameter)
{ if (1==option) document.getElementById('iFrameName_1').contentWindow
.functionInIFrame(parameter)
else document.getElementById('iFrameName_2').contentWindow
.functionInIFrame(parameter);
};
When “parameter” is reference to variable located parent window, things become little complex. JavaScript indeed regards it is about the variable in iFrame window. One needs to declare parameter locally first:
var parameter = parent.paraInParent;
What about in iFrame child window, calling corssWindow() function is through dynamic insertion? Something like:
window.onload = function()
{ document.getElementById('dynamicContent').innerHTML = '‹a href="#" onclick="parent.crossWindow(‘ + parameter + ‘)"›Click here to run function in parent window‹/a›';
};
No, it would never work properly if parameter is the reference referring back to another variable in parent window.
(To be continued)
Issues of Array in JavaScript
It is noticed the initialization of a variable when declared as an element of array in not allowed. For instance:
var arr = [1, 2, 3];
var num = arr[0];
Assigning value to variable num "num = arr[0]; " needs to be done separately.
Since JavaScript arrays are assigned by reference, when array to be passed across iFrame, an issue of speed was met, especially for Mozilla Firefox.
Scripts in iFrame page:
var newArray = parent.arr;
When calling newArray[0] immediate after above declaration, it often generates error of "newArray is not defined". HTML pages are actual independent programs (stateless), it is no wonder reference across programs facing such kind of problem. It is therefore suggested avoiding passing array across parent page with iFrame page. Alternatively, slice array into single dimensional variable will do.
The reason behind this issue is this syntax of passing value is also true for object reference passing through HTML pages. We know that the reference acts as pointer to point to original variable rather than make a copy, any using of the reference is indeed reference back to parent HTML's object. It does cause a complex process behind the scent.
For same reason, if one wants to turn array in JavaScript, the best approach is to turn it into a string first, then pass the string to php, then use explode() to turn it back to array in php.
http://www.webdeveloper.com/forum/archive/index.php/t-93920.html
var arr = [1, 2, 3];
var num = arr[0];
Assigning value to variable num "num = arr[0]; " needs to be done separately.
Since JavaScript arrays are assigned by reference, when array to be passed across iFrame, an issue of speed was met, especially for Mozilla Firefox.
Scripts in iFrame page:
var newArray = parent.arr;
When calling newArray[0] immediate after above declaration, it often generates error of "newArray is not defined". HTML pages are actual independent programs (stateless), it is no wonder reference across programs facing such kind of problem. It is therefore suggested avoiding passing array across parent page with iFrame page. Alternatively, slice array into single dimensional variable will do.
The reason behind this issue is this syntax of passing value is also true for object reference passing through HTML pages. We know that the reference acts as pointer to point to original variable rather than make a copy, any using of the reference is indeed reference back to parent HTML's object. It does cause a complex process behind the scent.
For same reason, if one wants to turn array in JavaScript, the best approach is to turn it into a string first, then pass the string to php, then use explode() to turn it back to array in php.
http://www.webdeveloper.com/forum/archive/index.php/t-93920.html
iFrame Height Issue
Changing height of iFrame from JavaScript function becomes painful task in new versions of both IE and Firefox. However, if one uses iFrame, adjusting its height to fit its contents is essential. The problem is the height of content is unknown when dramatic feeding required. The “orthodox” method to accomplish this task is to open iFrame with any height first, then adjust it on onLoad event. Width is less painful because for those iFrame to fit complete screen width, width=“100%” can be defined when iFrame is defined.
Basic idea of this “orthodox” approach includes two steps, detect the correct height and resize to it.
Detecting methods include:
- document.documentElement.clientHeight
- contentWindow.document.body.scrollHeight
Resize method is:
- document.getElementById('myIframe').style.height = newHeight
Basically, this approach does not work. There are several problems around it, such as assignment to .style.height does not work in Firefox. More serious problem is contentWindow.document.body.scrollHeight will return permission denial error message if host page and iFrame content page are not in the same domain; more precisely, the scheme, hostname and port match.
Another approach is to find the correct height before opening the iFrame. It is hard to detect the height of page to be inside of iFrame. However, since iFrame can have its own scroll bar, it may not be necessary to detect content’s height. The best approach might be to find available screen height of user after your title head. Following methods have been tested in Firefox and IE:
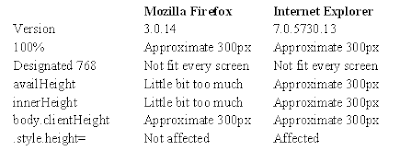
It appears Internet Explorer does not response to any of detections.
Following is the codes to apply this approach:
Codes in head:
‹script type="text/javascript"›
var url = "http://www.google.com/" // URL for your iFrame.
var titleHeight = 240;
var frameHeight = Math.max((screen.availHeight ? (screen.availHeight-titleHeight) : (window.innerHeight ? (window.innerHeight-titleHeight) : document.body.clientHeight)), 768);
window.document.onload = function {document.getElementById('linkIframe').style.height = frameHeight};
‹/script›
Codes in body:
‹script type="text/javascript"›
document.write('‹iframe id="linkIframe" width="100%" height="' + frameHeight + '" src="' + url + '" name="content"›‹/iframe›');
‹/script›
Basic idea of this “orthodox” approach includes two steps, detect the correct height and resize to it.
Detecting methods include:
- document.documentElement.clientHeight
- contentWindow.document.body.scrollHeight
Resize method is:
- document.getElementById('myIframe').style.height = newHeight
Basically, this approach does not work. There are several problems around it, such as assignment to .style.height does not work in Firefox. More serious problem is contentWindow.document.body.scrollHeight will return permission denial error message if host page and iFrame content page are not in the same domain; more precisely, the scheme, hostname and port match.
Another approach is to find the correct height before opening the iFrame. It is hard to detect the height of page to be inside of iFrame. However, since iFrame can have its own scroll bar, it may not be necessary to detect content’s height. The best approach might be to find available screen height of user after your title head. Following methods have been tested in Firefox and IE:
It appears Internet Explorer does not response to any of detections.
Following is the codes to apply this approach:
Codes in head:
‹script type="text/javascript"›
var url = "http://www.google.com/" // URL for your iFrame.
var titleHeight = 240;
var frameHeight = Math.max((screen.availHeight ? (screen.availHeight-titleHeight) : (window.innerHeight ? (window.innerHeight-titleHeight) : document.body.clientHeight)), 768);
window.document.onload = function {document.getElementById('linkIframe').style.height = frameHeight};
‹/script›
Codes in body:
‹script type="text/javascript"›
document.write('‹iframe id="linkIframe" width="100%" height="' + frameHeight + '" src="' + url + '" name="content"›‹/iframe›');
‹/script›
Clearing Obstacles for MS Excel Application Upgrading to 2007
,Recently an Excel application wrote in 2003 version needs to be converted into 2007 following the network infrastructure upgrade. It is noticed there are three parts needed to be attended.
In VBA micro of old application, value of attribute FileFormat for ActiveWorkbook.SaveAs was xlNormal. It is no longer allowed in Excel 2007. The FileFormat now needs to be explicitly expressed. Common values are:
51 = xlOpenXMLWorkbook (without macro's in 2007, xlsx)
52 = xlOpenXMLWorkbookMacroEnabled (with or without macro's in 2007, xlsm)
50 = xlExcel12 (Excel Binary Workbook in 2007 with or without macro's, xlsb)
56 = xlExcel8 (97-2003 format in Excel 2007, xls)
In this case, value of 52 is used. For more information, please refer to http://www.rondebruin.nl/saveas.htm.
File name is also an issue. This application automatically archives data everyday into a file, of which there is a space in file’s name. Now VBA in Excel 2007 converted the space to ‘%’ sign. Accordingly, the archiving file’s name needs to be changed, as well as the file type changed from .xls to .xlsm.
Originally archiving function involved large chunk of cells being copied from one worksheet to another. After conversion to Excel 2007, it is noticed the file’s size increase drastically without apparent reason. The educated guessing is somehow the application accumulated its clipboard during the automatic archiving process which involved copy-paste and save-as. However, nothing was found either in worksheets or clipboard. Web search shows no such error had been reported to Microsoft. After rewrote codes in this part to remove format as part of clipboard, the problem is resolved, although the reason is still not be found.
In VBA micro of old application, value of attribute FileFormat for ActiveWorkbook.SaveAs was xlNormal. It is no longer allowed in Excel 2007. The FileFormat now needs to be explicitly expressed. Common values are:
51 = xlOpenXMLWorkbook (without macro's in 2007, xlsx)
52 = xlOpenXMLWorkbookMacroEnabled (with or without macro's in 2007, xlsm)
50 = xlExcel12 (Excel Binary Workbook in 2007 with or without macro's, xlsb)
56 = xlExcel8 (97-2003 format in Excel 2007, xls)
In this case, value of 52 is used. For more information, please refer to http://www.rondebruin.nl/saveas.htm.
File name is also an issue. This application automatically archives data everyday into a file, of which there is a space in file’s name. Now VBA in Excel 2007 converted the space to ‘%’ sign. Accordingly, the archiving file’s name needs to be changed, as well as the file type changed from .xls to .xlsm.
Originally archiving function involved large chunk of cells being copied from one worksheet to another. After conversion to Excel 2007, it is noticed the file’s size increase drastically without apparent reason. The educated guessing is somehow the application accumulated its clipboard during the automatic archiving process which involved copy-paste and save-as. However, nothing was found either in worksheets or clipboard. Web search shows no such error had been reported to Microsoft. After rewrote codes in this part to remove format as part of clipboard, the problem is resolved, although the reason is still not be found.
Clearing Obstacles for Automation in MS Access
There are many obstructive dialogue windows require manual response, which does really slow down the automation process. In one special case, MS Access is used to construct and update a considerable huge data warehouse. The update automation macro consists of over a hundred queries. The dialogue windows effectively destroy the automation itself.
Many of these dialogue windows can be set in Access Options. However, a special window cannot be resolved through setting. The message is “There isn't enough disk space or memory to undo the data changes this action query is about to make.” This is caused by running action queries on a large table.
Support of Microsoft regards this as an error message: http://support.microsoft.com/kb/161329, which also provides a number of solutions. Method 3, Setting the UseTransaction Property in an Action Query is particularily helpful:
If a stored action query causes the error, you can set its UseTransaction property to No. Note that if you do this, you will not able to roll back your changes if there is a problem or an error while the query is executing:
1. Open the query in Design view.
2. On the View menu, click Properties.
3. Click an empty space in the upper half of the query window to display the Query Properties dialog box.
4. Set the UseTransaction property to No.
5. Save the query and close it.
Most important, you would need to set no warnings, as follows:
1. In your first Macro row, right click mouse to insert a new row.
2. Click "Show All Actions" in Show/Hide menu.
3. In Action of your inserted first row, select SetWarnings.
4. Change Arguments value of the first row to "No".
5. Click Save.
Now you are ok to run Macros without any warning.
Many of these dialogue windows can be set in Access Options. However, a special window cannot be resolved through setting. The message is “There isn't enough disk space or memory to undo the data changes this action query is about to make.” This is caused by running action queries on a large table.
Support of Microsoft regards this as an error message: http://support.microsoft.com/kb/161329, which also provides a number of solutions. Method 3, Setting the UseTransaction Property in an Action Query is particularily helpful:
If a stored action query causes the error, you can set its UseTransaction property to No. Note that if you do this, you will not able to roll back your changes if there is a problem or an error while the query is executing:
1. Open the query in Design view.
2. On the View menu, click Properties.
3. Click an empty space in the upper half of the query window to display the Query Properties dialog box.
4. Set the UseTransaction property to No.
5. Save the query and close it.
Most important, you would need to set no warnings, as follows:
1. In your first Macro row, right click mouse to insert a new row.
2. Click "Show All Actions" in Show/Hide menu.
3. In Action of your inserted first row, select SetWarnings.
4. Change Arguments value of the first row to "No".
5. Click Save.
Now you are ok to run Macros without any warning.
A Good IT Certification Study Website
IT Exams is very good IT certification study website, if not the best. It links to a bunch of text books and dump tests, which are complete free. Unfortunately, it only has Java and Oracle certification information.
IT Exams' address is http://itexams.weebly.com/.
IT Exams' address is http://itexams.weebly.com/.
Temporarily Disable and Re-enable the Constraints in Oracle
SQL command script files to disable and enable all constraints:
Disable:
set feedback off
set verify off
set echo off
prompt Finding constraints to disable...
set termout off
set pages 80
set heading off
set linesize 120
spool tmp_disable.sql
select 'spool igen_disable.log;' from dual;
select 'ALTER TABLE '||substr(c.table_name,1,35)||' DISABLE CONSTRAINT '||constraint_name||' ;'
from user_constraints c join user_tables u on c.table_name = u.table_name;
select 'exit;' from dual;
set termout on
prompt Disabling constraints now...
set termout off
@tmp_disable.sql;
exit
/
Enable:
set feedback off
set verify off
set wrap off
set echo off
prompt Finding constraints to enable...
set termout off
set lines 120
set heading off
spool tmp_enable.sql
select 'spool igen_enable.log;' from dual;
select 'ALTER TABLE '||substr(c.table_name,1,35)||' ENABLE CONSTRAINT '||constraint_name||' ;'
from user_constraints c join user_tables u on c.table_name = u.table_name;
/
select 'exit;' from dual;
set termout on
prompt Enabling constraints now...
set termout off
@tmp_enable;
!rm -i tmp_enable.sql;
exit
/
Scripts in PL/SQL to disable and enable all constraints:
Disable:
BEGIN
FOR i IN
( SELECT c.owner
, c.table_name
, c.constraint_name
FROM user_constraints c
JOIN user_tables t ON c.table_name = t.table_name
WHERE c.status = 'ENABLED'
ORDER BY c.constraint_type DESC
)
LOOP
dbms_utility.exec_ddl_statement('alter table ' || i.owner || '.' || i.table_name || ' disable constraint ' || i.constraint_name);
END LOOP;
END;
/
Enable:
BEGIN
FOR i IN
( SELECT c.owner
, c.table_name
, c.constraint_name
FROM user_constraints c
JOIN user_tables t ON c.table_name = t.table_name
WHERE c.status = 'DISABLED'
ORDER BY c.constraint_type
)
LOOP
dbms_utility.exec_ddl_statement('alter table ' || c.owner || '.' || c.table_name || ' enable constraint ' || c.constraint_name);
END LOOP;
END;
/
Disable:
set feedback off
set verify off
set echo off
prompt Finding constraints to disable...
set termout off
set pages 80
set heading off
set linesize 120
spool tmp_disable.sql
select 'spool igen_disable.log;' from dual;
select 'ALTER TABLE '||substr(c.table_name,1,35)||' DISABLE CONSTRAINT '||constraint_name||' ;'
from user_constraints c join user_tables u on c.table_name = u.table_name;
select 'exit;' from dual;
set termout on
prompt Disabling constraints now...
set termout off
@tmp_disable.sql;
exit
/
Enable:
set feedback off
set verify off
set wrap off
set echo off
prompt Finding constraints to enable...
set termout off
set lines 120
set heading off
spool tmp_enable.sql
select 'spool igen_enable.log;' from dual;
select 'ALTER TABLE '||substr(c.table_name,1,35)||' ENABLE CONSTRAINT '||constraint_name||' ;'
from user_constraints c join user_tables u on c.table_name = u.table_name;
/
select 'exit;' from dual;
set termout on
prompt Enabling constraints now...
set termout off
@tmp_enable;
!rm -i tmp_enable.sql;
exit
/
Scripts in PL/SQL to disable and enable all constraints:
Disable:
BEGIN
FOR i IN
( SELECT c.owner
, c.table_name
, c.constraint_name
FROM user_constraints c
JOIN user_tables t ON c.table_name = t.table_name
WHERE c.status = 'ENABLED'
ORDER BY c.constraint_type DESC
)
LOOP
dbms_utility.exec_ddl_statement('alter table ' || i.owner || '.' || i.table_name || ' disable constraint ' || i.constraint_name);
END LOOP;
END;
/
Enable:
BEGIN
FOR i IN
( SELECT c.owner
, c.table_name
, c.constraint_name
FROM user_constraints c
JOIN user_tables t ON c.table_name = t.table_name
WHERE c.status = 'DISABLED'
ORDER BY c.constraint_type
)
LOOP
dbms_utility.exec_ddl_statement('alter table ' || c.owner || '.' || c.table_name || ' enable constraint ' || c.constraint_name);
END LOOP;
END;
/
Software Patch Installation Batch Files
The example here is to install an Oracle database application patch. The basic structure of patch installation scripts shall include two folders and two files:
Folder 1: Logs
Folder 2: Scripts
File 1: Readme.txt
File 2: Setup.bat (or other name)
Of course, there would be some SQL script files in Scripts folder. Log files in Logs folder would be generated by these script files in Scripts folder. The working procedure is to run installer batch file (here Setup.bat), which will call other batch files (we do not have here) and/or script files stored in Scripts folder. In there example, the batch file open sqlplus.exe followed by user id, password and the SQL scripts (in file), or package to run.
Following is the example of batch file:
@echo off
:: ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
:: PLEASE REVIEW AND ADJUST ENVIRONMENT VARIABLES BETWEEN < >
:: ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
CLS
set NETALIAS=
set USERID=
set PASSWORD=
set COMMONID=
set COMPASS=
:: ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
:: ORACLE_VERSION are 9.2 (for 9i) or 10.2.0 (for 10g)
:: ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
set ORACLE_VERSION=
set ORACLE_HOME=C:\oracle\product\%ORACLE_VERSION%\db_1
set SQLUTIL=%ORACLE_HOME%\bin\sqlplus.exe
set FOLDERPATH=%cd%
echo #####################################################
echo.
echo You are just about to run SCRAMBLING SCRIPT on database
echo.
echo [**** %NETALIAS% ****]
echo.
echo Schema
echo.
echo [**** %USERID% ****]
echo.
echo If this is not correct Database/Schema then
echo.
echo press CTL + C to cancel the script.....
echo.
echo #####################################################
pause
"%SQLUTIL%" %USERID%/%PASSWORD%@%NETALIAS% @"%FOLDERPATH%\Scripts\Truncate.sql"
"%SQLUTIL%" %USERID%/%PASSWORD%@%NETALIAS% @"%FOLDERPATH%\Scripts\Create_Tables.sql"
"%SQLUTIL%" %USERID%/%PASSWORD%@%NETALIAS% @"%FOLDERPATH%\Scripts\DATA_SCRAMBLE.spec"
"%SQLUTIL%" %USERID%/%PASSWORD%@%NETALIAS% @"%FOLDERPATH%\Scripts\DATA_SCRAMBLE.body"
"%SQLUTIL%" %USERID%/%PASSWORD%@%NETALIAS% @"%FOLDERPATH%\Scripts\Cleanup.sql"
pause
Note: NETALIAS, USERID following “set” commend are variables. %...% is the way to use these variables.
Following is the Truncate.sql:
PROMPT Truncating data. Please wait...
TRUNCATE TABLE BATCH_BUF$RECON$DETAIL;
TRUNCATE TABLE BATCH_BUF$VALU$SUMMARY_PEN;
-- Enable/Disable primary, unique and foreign key constraints alter table BATCH_BUF$VALU$SUMMARY_PEN disable constraint BATCH_BUF$VALU$SUMMARY_PEN$FK1;
EXIT;
Folder 1: Logs
Folder 2: Scripts
File 1: Readme.txt
File 2: Setup.bat (or other name)
Of course, there would be some SQL script files in Scripts folder. Log files in Logs folder would be generated by these script files in Scripts folder. The working procedure is to run installer batch file (here Setup.bat), which will call other batch files (we do not have here) and/or script files stored in Scripts folder. In there example, the batch file open sqlplus.exe followed by user id, password and the SQL scripts (in file), or package to run.
Following is the example of batch file:
@echo off
:: ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
:: PLEASE REVIEW AND ADJUST ENVIRONMENT VARIABLES BETWEEN < >
:: ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
CLS
set NETALIAS=
set USERID=
set PASSWORD=
set COMMONID=
set COMPASS=
:: ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
:: ORACLE_VERSION are 9.2 (for 9i) or 10.2.0 (for 10g)
:: ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
set ORACLE_VERSION=
set ORACLE_HOME=C:\oracle\product\%ORACLE_VERSION%\db_1
set SQLUTIL=%ORACLE_HOME%\bin\sqlplus.exe
set FOLDERPATH=%cd%
echo #####################################################
echo.
echo You are just about to run SCRAMBLING SCRIPT on database
echo.
echo [**** %NETALIAS% ****]
echo.
echo Schema
echo.
echo [**** %USERID% ****]
echo.
echo If this is not correct Database/Schema then
echo.
echo press CTL + C to cancel the script.....
echo.
echo #####################################################
pause
"%SQLUTIL%" %USERID%/%PASSWORD%@%NETALIAS% @"%FOLDERPATH%\Scripts\Truncate.sql"
"%SQLUTIL%" %USERID%/%PASSWORD%@%NETALIAS% @"%FOLDERPATH%\Scripts\Create_Tables.sql"
"%SQLUTIL%" %USERID%/%PASSWORD%@%NETALIAS% @"%FOLDERPATH%\Scripts\DATA_SCRAMBLE.spec"
"%SQLUTIL%" %USERID%/%PASSWORD%@%NETALIAS% @"%FOLDERPATH%\Scripts\DATA_SCRAMBLE.body"
"%SQLUTIL%" %USERID%/%PASSWORD%@%NETALIAS% @"%FOLDERPATH%\Scripts\Cleanup.sql"
pause
Note: NETALIAS, USERID following “set” commend are variables. %...% is the way to use these variables.
Following is the Truncate.sql:
PROMPT Truncating data. Please wait...
TRUNCATE TABLE BATCH_BUF$RECON$DETAIL;
TRUNCATE TABLE BATCH_BUF$VALU$SUMMARY_PEN;
-- Enable/Disable primary, unique and foreign key constraints alter table BATCH_BUF$VALU$SUMMARY_PEN disable constraint BATCH_BUF$VALU$SUMMARY_PEN$FK1;
EXIT;
A Privacy Issue for Google AdSense
Google AdSense allows readers to gain the Publisher ID through viewing web page's source code in HTML. This may lead to privacy leaking to some degree.
Here is a case to show how people can explore such public available information. Blog site blog.dwnews.com is a popular political forum amongst overseas Chinese communities. The owner of this forum tries to create a forum without bias and welcomes people having different options to debate in the forum. As the result, the debates are always so ferocious between groups of pro-democracy and pro- Chinese Government. In aims to avoid the possible trouble with Chinese Government, many bloggers are anonymous there.
One blogger through Google AdSense' publisher ID found out several popular blogs were actually run by a same person, or presumably the same person because the AdSense Publisher IDs for these blogs are the same. He attached accordingly based on his finding, followed by a series of incidents. At the end of it, one of them declared he leaves the site permanently, and the account of the other one, ranked number one in current activities, had been cancelled by the webmaster. What lucky here is, both of them did not reside in China and we did not see someone ends up in the jail.
That was in June 2009, two months ago.
Here is a case to show how people can explore such public available information. Blog site blog.dwnews.com is a popular political forum amongst overseas Chinese communities. The owner of this forum tries to create a forum without bias and welcomes people having different options to debate in the forum. As the result, the debates are always so ferocious between groups of pro-democracy and pro- Chinese Government. In aims to avoid the possible trouble with Chinese Government, many bloggers are anonymous there.
One blogger through Google AdSense' publisher ID found out several popular blogs were actually run by a same person, or presumably the same person because the AdSense Publisher IDs for these blogs are the same. He attached accordingly based on his finding, followed by a series of incidents. At the end of it, one of them declared he leaves the site permanently, and the account of the other one, ranked number one in current activities, had been cancelled by the webmaster. What lucky here is, both of them did not reside in China and we did not see someone ends up in the jail.
That was in June 2009, two months ago.
A Practical Case of Transpose Query
Let us say a pension fund wants to generate a summary consists of total contributions had been made so far for each member, by their employers. The fund does not care about exact contribution made by each employers for each member. However, the fund wants to know the three employers paid the contributions most for each member. As the result, the summary table should have 5 columns: Member_Id (unique), Total_Contributions, Employer_1, Employer_2, Employer_3. If a member has less than three employers, leave it blank. Each member should have occupied one row only.
Simple SQL query of SELECT GROUP BY won't be able to accomplish this task, since it is a transpose requirement, namely, turning the row into column. However, CASE or DECODE in PL/SQL will do. The equivalent of CASE in Microsoft Office Access is IIF. Alternatively, TRANSFORM in Access and SELECT PIVOT in PL/SQL can be used. By use CASE or DECODE, it is possible to avoid the complex programming. There are five steps:
1. To get dataset first which should consist of all necessary information. Let us use Dataset_All to describe it:
SELECT Member_Id
, Employer_Id
, SUM(Contribution) Total_Contribution
FROM Tab
GROUP BY Member_Id
, Employer_Id
ORDER BY Member_Id
ORDER BY is important since it would make sure the ROWNUM is in align with the Member_Id.
2. Get a dataset which consists of the Member_Id and their last ROWNUM in Dataset_All. Let us name it Dataset_Uni:
SELECT Member_Id
, MAX(ROWNUM) Serial_No
FROM Dataset_All
GROUP BY Member_Id
Using GROUP BY to get largest ROWNUM if same Member_Id having multiple rows.
3. To flag each employer according their rank, start from 1. Let us name it Dataset_Rank:
SELECT Dataset_Uni.Member_Id
, Total_Contribution
, (Serial_No - Dataset_All.ROWNUM + 1) Employer_Serial
, Employer_Id
FROM Dataset_Uni
JOIN Dataset_All ON Dataset_Uni.Member_Id = Dataset_All.Member_Id
4. Transpose process. We also use the numerical feather of Employer_Id which was generated from sequence:
SELECT Member_Id
, Total_Contribution
, SUM(CASE Employer_Serial (WHEN 1 Employer_Id, ELSE 0)) Employer_1
, SUM(CASE Employer_Serial (WHEN 2 Employer_Id, ELSE 0)) Employer_2
, SUM(CASE Employer_Serial (WHEN 3 Employer_Id, ELSE 0)) Employer_3
FROM Dataset_Rank
GROUP BY Member_Id
, Total_Contribution
ORDER BY Member_Id
Put everything together:
WITH Dataset_All AS
( SELECT Member_Id
, Employer_Id
, SUM(Contribution) Total_Contributio
FROM Tab
GROUP BY Member_Id
, Employer_Id
ORDER BY Member_Id
)
SELECT Member_Id
, Total_Contribution
, SUM(CASE Employer_Serial (WHEN 1 Employer_Id, ELSE 0)) Employer_1
, SUM(CASE Employer_Serial (WHEN 2 Employer_Id, ELSE 0)) Employer_2
, SUM(CASE Employer_Serial (WHEN 3 Employer_Id, ELSE 0)) Employer_3
FROM
( SELECT Dataset_Uni.Member_Id
, Total_Contribution
, (Serial_No - Dataset_All.ROWNUM + 1) Employer_Serial
, Employer_Id
FROM
( SELECT Member_Id
, MAX(ROWNUM) Serial_No
FROM Dataset_All
GROUP BY Member_Id
) Dataset_Uni
JOIN Dataset_All ON Dataset_Uni.Member_Id = Dataset_All.Member_Id
)
GROUP BY Member_Id
, Total_Contribution
ORDER BY Member_Id
Done.
Simple SQL query of SELECT GROUP BY won't be able to accomplish this task, since it is a transpose requirement, namely, turning the row into column. However, CASE or DECODE in PL/SQL will do. The equivalent of CASE in Microsoft Office Access is IIF. Alternatively, TRANSFORM in Access and SELECT PIVOT in PL/SQL can be used. By use CASE or DECODE, it is possible to avoid the complex programming. There are five steps:
1. To get dataset first which should consist of all necessary information. Let us use Dataset_All to describe it:
SELECT Member_Id
, Employer_Id
, SUM(Contribution) Total_Contribution
FROM Tab
GROUP BY Member_Id
, Employer_Id
ORDER BY Member_Id
ORDER BY is important since it would make sure the ROWNUM is in align with the Member_Id.
2. Get a dataset which consists of the Member_Id and their last ROWNUM in Dataset_All. Let us name it Dataset_Uni:
SELECT Member_Id
, MAX(ROWNUM) Serial_No
FROM Dataset_All
GROUP BY Member_Id
Using GROUP BY to get largest ROWNUM if same Member_Id having multiple rows.
3. To flag each employer according their rank, start from 1. Let us name it Dataset_Rank:
SELECT Dataset_Uni.Member_Id
, Total_Contribution
, (Serial_No - Dataset_All.ROWNUM + 1) Employer_Serial
, Employer_Id
FROM Dataset_Uni
JOIN Dataset_All ON Dataset_Uni.Member_Id = Dataset_All.Member_Id
4. Transpose process. We also use the numerical feather of Employer_Id which was generated from sequence:
SELECT Member_Id
, Total_Contribution
, SUM(CASE Employer_Serial (WHEN 1 Employer_Id, ELSE 0)) Employer_1
, SUM(CASE Employer_Serial (WHEN 2 Employer_Id, ELSE 0)) Employer_2
, SUM(CASE Employer_Serial (WHEN 3 Employer_Id, ELSE 0)) Employer_3
FROM Dataset_Rank
GROUP BY Member_Id
, Total_Contribution
ORDER BY Member_Id
Put everything together:
WITH Dataset_All AS
( SELECT Member_Id
, Employer_Id
, SUM(Contribution) Total_Contributio
FROM Tab
GROUP BY Member_Id
, Employer_Id
ORDER BY Member_Id
)
SELECT Member_Id
, Total_Contribution
, SUM(CASE Employer_Serial (WHEN 1 Employer_Id, ELSE 0)) Employer_1
, SUM(CASE Employer_Serial (WHEN 2 Employer_Id, ELSE 0)) Employer_2
, SUM(CASE Employer_Serial (WHEN 3 Employer_Id, ELSE 0)) Employer_3
FROM
( SELECT Dataset_Uni.Member_Id
, Total_Contribution
, (Serial_No - Dataset_All.ROWNUM + 1) Employer_Serial
, Employer_Id
FROM
( SELECT Member_Id
, MAX(ROWNUM) Serial_No
FROM Dataset_All
GROUP BY Member_Id
) Dataset_Uni
JOIN Dataset_All ON Dataset_Uni.Member_Id = Dataset_All.Member_Id
)
GROUP BY Member_Id
, Total_Contribution
ORDER BY Member_Id
Done.
Koncord Applied Excel Functions
We have uploaded some applied Excel functions. These are some basic functions, but very much useful in day-to-day working. This Excel file include some functions of string parsing, dates, telephone number parsing, and coordinates parsing.
Following is the link:
Koncord Applied Excel Functions
Following is the Terms of Services of Koncord Partners: http://koncordpartners.blogspot.com/2010/06/terms-of-services.html
Following is the link:
Koncord Applied Excel Functions
Following is the Terms of Services of Koncord Partners: http://koncordpartners.blogspot.com/2010/06/terms-of-services.html
Launch Koncord Homepage Online
We launched two size of Koncord Homepage online. Address is as follows:
Koncord Homepage
All you need to do is just set it as your homepage for your browsers.
Koncord Homepage
All you need to do is just set it as your homepage for your browsers.
Local Address Error in HTML for Firefox
If you include the address like this “C:\Documents and Settings\...” in your HTML page, it would be Ok for IE to open it. However, it would be a problem for Firefox, sometimes. Following is the error message:
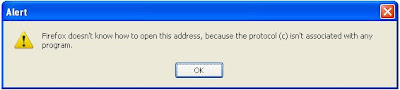
There is easy way to fix it – simply add “file:\\\” in front of your local address. It would look like: "file:\\\C:\Documents and Settings\..."
http://www.codingforums.com/archive/index.php/t-53382.html
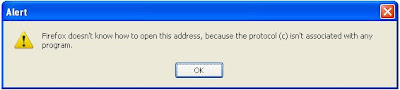
There is easy way to fix it – simply add “file:\\\” in front of your local address. It would look like: "file:\\\C:\Documents and Settings\..."
http://www.codingforums.com/archive/index.php/t-53382.html
First Beta Version of Koncord Homepage Is Free to Download
We have prepared a single HTML page which can be used as a Homepage for your browsers. This page consists of popular websites, such as Gmail, Hotmail, etc. Of course, it also has built-in Koncord Private Search Engine.
This homepage is just like favourites in IE and bookmarks in Firefox.
Here is free download site:
Download from Uploading.com
The address is: http://uploading.com/files/7Z17FDD2/Koncord.html.html
This homepage is just like favourites in IE and bookmarks in Firefox.
Here is free download site:
Download from Uploading.com
The address is: http://uploading.com/files/7Z17FDD2/Koncord.html.html
About ISO Image
An ISO image is an archive file (also known as a disk image) of an CD/DVD in a format defined by the International Organization for Standardization (ISO).
So, it is a virtual CD. There are three actions in relation to ISO image file.
1. Archiving a file or a folder into an ISO image file. This some times called create an ISO image file, or burn into an ISO image. The source files can be a CD/DVD as well. Currently many software installer directly accept this kind of virtual CD. For instance, installing an Linux OS on VMware Server, the VMware Server would prefer you have .iso files.
2. Turn ISO image back to real CD/DVD. Some time this procedure called burn an ISO image, burn an ISO CD/DVD, burn ISO image into CD/DVD.
3. Using or reading ISO image file. When you use an real CD/DVD, you would need a CD/DVD ROM Drive. So, to use ISO image file, the virtual CD/DVD, you will need a virtual CD ROM too. It is also called mount an ISO image, mount ISO file, etc.
Many times, verbs Create, Burn and Mount are interchangeable. It just create confusion what they are talking about. Software of ISO Image may not having all of these three functions. Installer software of course covers the third function mentioned above. So, if you have software installation files already sit in your hard disk, and want to install it, but your installer software could only accept the ISO or CD (this process sometimes called “network installation”), what you got to do?
Option A: Burn your files into a CD.
Option B: Archive your files into a ISO image file. The best software for this purpose is Folder2Iso at http://depositfiles.com/en/files/789051.
http://en.wikipedia.org/wiki/.iso
http://www.online-tech-tips.com/computer-tips/how-to-create-mount-and-burn-iso-image-files-for-free/
http://www.petri.co.il/mount_iso_files_in_windows_vista.htm
http://en.wikipedia.org/wiki/List_of_ISO_image_software
So, it is a virtual CD. There are three actions in relation to ISO image file.
1. Archiving a file or a folder into an ISO image file. This some times called create an ISO image file, or burn into an ISO image. The source files can be a CD/DVD as well. Currently many software installer directly accept this kind of virtual CD. For instance, installing an Linux OS on VMware Server, the VMware Server would prefer you have .iso files.
2. Turn ISO image back to real CD/DVD. Some time this procedure called burn an ISO image, burn an ISO CD/DVD, burn ISO image into CD/DVD.
3. Using or reading ISO image file. When you use an real CD/DVD, you would need a CD/DVD ROM Drive. So, to use ISO image file, the virtual CD/DVD, you will need a virtual CD ROM too. It is also called mount an ISO image, mount ISO file, etc.
Many times, verbs Create, Burn and Mount are interchangeable. It just create confusion what they are talking about. Software of ISO Image may not having all of these three functions. Installer software of course covers the third function mentioned above. So, if you have software installation files already sit in your hard disk, and want to install it, but your installer software could only accept the ISO or CD (this process sometimes called “network installation”), what you got to do?
Option A: Burn your files into a CD.
Option B: Archive your files into a ISO image file. The best software for this purpose is Folder2Iso at http://depositfiles.com/en/files/789051.
http://en.wikipedia.org/wiki/.iso
http://www.online-tech-tips.com/computer-tips/how-to-create-mount-and-burn-iso-image-files-for-free/
http://www.petri.co.il/mount_iso_files_in_windows_vista.htm
http://en.wikipedia.org/wiki/List_of_ISO_image_software
Another Urban Legend?
Just heard a story. A guy bought a desktop of the most popular PC brand with a most popular PC operating system, a year ago. The OS’s version is that has a very bad reputation, you know. He found a “black process” or processes run on his PC, which has following features:
1. The process does not registered in Task Manager, neither in CPU nor in Memory.
2. It runs regularly every day from around 1:00 am, and stops in next morning. However, every Saturday is bad. It runs until around 1:00 pm, sometime last to 8:00 pm. It does not start or end at exactly same time everyday. You would see this speed makes the PC completely useless.
3. It occupies huge amount of CPU and Memory, which makes a noticeable and considerable slow of response from PC. For instance, Ctrl + Alt + Del may take up to five minutes to enable Task Manager appears. The total RAM is 2G. When black process running and when no other applications opens (just re-started PC), Task Manager shows total 2% being used and around 20% Memory being used, yet, the PC is as slow as any mouse movement can take 2 minutes.
4. Total amount of 50G Hard Disk space could not be accounted for, given the fact of total 110G Hard Disk space has been used including all hidden files and recycle bin. When black process runs, the Hard Disk is flashing. The total Hard Disk space if around 460G for this driver (C:\).
5. Black process running does not require the Internet connection.
6. Black process runs before user logins into OS. Restarting computer, either softly or hardly does not stop its running. It has been noticed Safe Mode could not stop it either.
7. Do not know if it was caused by the black process or system bug, when black process runs, SOMETIMES Ctrl + Alt + Del generate an error message in pop-up window: “Unable to create a security option. Login failed”.
8. It was believed as virus infection. However, he failed to find out if this contention can be confirmed after he tried much anti-virus software.
9. No harm has been noticed apart from the slow response from PC.
10. It has been confirmed this black process is not any of the scheduled system maintenance.
Based on these limited information, it is hard to make conclusion. However, it looks like a system bug or an urban legend – someone is conducting a secret SETI@home like distributed computing scheme.
http://en.wikipedia.org/wiki/SETI@home
1. The process does not registered in Task Manager, neither in CPU nor in Memory.
2. It runs regularly every day from around 1:00 am, and stops in next morning. However, every Saturday is bad. It runs until around 1:00 pm, sometime last to 8:00 pm. It does not start or end at exactly same time everyday. You would see this speed makes the PC completely useless.
3. It occupies huge amount of CPU and Memory, which makes a noticeable and considerable slow of response from PC. For instance, Ctrl + Alt + Del may take up to five minutes to enable Task Manager appears. The total RAM is 2G. When black process running and when no other applications opens (just re-started PC), Task Manager shows total 2% being used and around 20% Memory being used, yet, the PC is as slow as any mouse movement can take 2 minutes.
4. Total amount of 50G Hard Disk space could not be accounted for, given the fact of total 110G Hard Disk space has been used including all hidden files and recycle bin. When black process runs, the Hard Disk is flashing. The total Hard Disk space if around 460G for this driver (C:\).
5. Black process running does not require the Internet connection.
6. Black process runs before user logins into OS. Restarting computer, either softly or hardly does not stop its running. It has been noticed Safe Mode could not stop it either.
7. Do not know if it was caused by the black process or system bug, when black process runs, SOMETIMES Ctrl + Alt + Del generate an error message in pop-up window: “Unable to create a security option. Login failed”.
8. It was believed as virus infection. However, he failed to find out if this contention can be confirmed after he tried much anti-virus software.
9. No harm has been noticed apart from the slow response from PC.
10. It has been confirmed this black process is not any of the scheduled system maintenance.
Based on these limited information, it is hard to make conclusion. However, it looks like a system bug or an urban legend – someone is conducting a secret SETI@home like distributed computing scheme.
http://en.wikipedia.org/wiki/SETI@home
Encoding of Chinese Characters
In computer text applications, the GB encoding scheme most often renders simplified Chinese characters, while Big5 most often renders traditional characters. Although neither encoding has an explicit connection with a specific character set, the lack of a one-to-one mapping between the simplified and traditional sets established a de facto linkage.
Since simplified Chinese conflated many characters into one and since the initial version of the GB encoding scheme, known as GB2312-80, contained only one code point for each character, it is impossible to use GB2312 to map to the bigger set of traditional characters. It is theoretically possible to use Big5 code to map to the smaller set of simplified character glyphs, although there is little market for such a product. Newer and alternative forms of GB have support for traditional characters. In particular, mainland authorities have now established GB 18030 as the official encoding standard for use in all mainland software publications. The encoding contains all East Asian characters included in Unicode 3.0. As such, GB 18030 encoding contains both simplified and traditional characters found in Big-5 and GB, as well as all characters found in Japanese and Korean encodings.
Unicode deals with the issue of simplified and traditional characters as part of the project of Han unification by including code points for each. This was rendered necessary by the fact that the linkage between simplified characters and traditional characters is not one-to-one. While this means that a Unicode system can display both simplified and traditional characters, it also means that different localization files are needed for each type.
The Chinese characters used in modern Japanese have also undergone simplification, but generally to a lesser extent than with simplified Chinese, it's worth mentioning that Japanese writing system reduced the number of Chinese characters in daily use, which was also part of the Japanese language reforms, thus, a number of complex characters were written phonetically. Reconciling these different character sets in Unicode became part of the controversial process of Han unification. Not surprisingly, some of the Chinese characters used in Japan are neither 'traditional' nor 'simplified'. In this case, these characters cannot be found in traditional/simplified Chinese dictionaries.
As a conclusion, GB18030 is the better choice for both Simplified Chinese and Traditional Chinese. Accordingly, in Google's language settings, zh-Hans/lang_zh_Hans is for Simplified Chinese and zh-Hant/lang_zh_Hant is for Traditional Chinese.
http://en.wikipedia.org/wiki/Simplified_Chinese_characters
http://www.google.com/coop/docs/cse/resultsxml.html#chineseSearch
Since simplified Chinese conflated many characters into one and since the initial version of the GB encoding scheme, known as GB2312-80, contained only one code point for each character, it is impossible to use GB2312 to map to the bigger set of traditional characters. It is theoretically possible to use Big5 code to map to the smaller set of simplified character glyphs, although there is little market for such a product. Newer and alternative forms of GB have support for traditional characters. In particular, mainland authorities have now established GB 18030 as the official encoding standard for use in all mainland software publications. The encoding contains all East Asian characters included in Unicode 3.0. As such, GB 18030 encoding contains both simplified and traditional characters found in Big-5 and GB, as well as all characters found in Japanese and Korean encodings.
Unicode deals with the issue of simplified and traditional characters as part of the project of Han unification by including code points for each. This was rendered necessary by the fact that the linkage between simplified characters and traditional characters is not one-to-one. While this means that a Unicode system can display both simplified and traditional characters, it also means that different localization files are needed for each type.
The Chinese characters used in modern Japanese have also undergone simplification, but generally to a lesser extent than with simplified Chinese, it's worth mentioning that Japanese writing system reduced the number of Chinese characters in daily use, which was also part of the Japanese language reforms, thus, a number of complex characters were written phonetically. Reconciling these different character sets in Unicode became part of the controversial process of Han unification. Not surprisingly, some of the Chinese characters used in Japan are neither 'traditional' nor 'simplified'. In this case, these characters cannot be found in traditional/simplified Chinese dictionaries.
As a conclusion, GB18030 is the better choice for both Simplified Chinese and Traditional Chinese. Accordingly, in Google's language settings, zh-Hans/lang_zh_Hans is for Simplified Chinese and zh-Hant/lang_zh_Hant is for Traditional Chinese.
http://en.wikipedia.org/wiki/Simplified_Chinese_characters
http://www.google.com/coop/docs/cse/resultsxml.html#chineseSearch
Why You Need a Private Search Engine
By create your own private search engine, you can put all your regular visiting websites into a single interface html page, then set it as your homepage. If you like, you can even set automatic login by a single click.
http://esupport.icewarp.com/index.php?_m=knowledgebase&_a=viewarticle&kbarticleid=51
http://esupport.icewarp.com/index.php?_m=knowledgebase&_a=viewarticle&kbarticleid=51
Set Language in Private Search Engine
Our previous posting Create Your Own Search Engine (http://koncordpartners.blogspot.com/2009/07/create-your-own-search-engine.html) could only display search results in English. Actually, the codes in that posting only have 4 parameters, you can add much more to suit your own needs. These 4 Google WebSearch Query Parameters are:
cx - Required. The cx parameter specifies a unique code that identifies a custom search engine. You must specify a Custom Search Engine using the cx parameter to retrieve search results from that CSE.
ie - Optional. The ie parameter sets the character encoding scheme that should be used to interpret the query string. The default ie value is latin1.
q - Optional. The q parameter specifies the search query entered by the user. Even though this parameter is optional, you must specify a value for at least one of the query parameters (as_epq, as_lq, as_oq, as_q, as_rq) to get search results.
sa - Required. It is indeed you submit the search query to Google search engine.
Today, we add three more parameters to show how the Simplified Chinese and Traditional Chinese can be interchangeable as search result.
hl - Optional. The hl parameter specifies the interface language (host language) of your user interface. To improve the performance and the quality of your search results, you are strongly encouraged to set this parameter explicitly. However, a successful search engine does not rely only on the parameters, but also settings of search engine itself. That is why you may also need your own account. “Site language”, is not only the site language, but also the search engine host language (the display language other than search result). Naturally, it should be set as English. “Your site encoding”, which is the searching language, should be GB18030 in this example.
lr - Optional. The lr (language restrict) parameter restricts search results to documents written in a particular language. Google WebSearch determines the language of a document by analyzing:
- the top-level domain (TLD) of the document's URL
- language meta tags within the document
- the primary language used in the body text of the document
c2coff - Optional. The c2coff parameter enables or disables the Simplified and Traditional Chinese Search feature. Please note, this parameter is only related to Chinese. For other language, no need to use this. The default value for this parameter is "0" (zero), meaning that the feature is enabled. Values for the c2coff parameter are: 1 is disabled, and 0 is enabled.
Following is codes with these two parameters. It means the search will be restricted to only websites wrote in three languages: US English, Simplified Chinese and Traditional Chinese.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en">
<head>
<title>
This is private search engine using Google facility
</title>
</head>
<body>
</br>
</br>
</br>
</br>
</br>
</br>
</br>
</br>
<form id="cse-search-box" action="http://www.google.com/cse">
<input type="hidden" value="partner-pub-3597878264183301:140hrt-vklq" name="cx">
<input type="hidden" value="GB18030" name="ie">
<input type="hidden" value="lang_en|lang_zh-Hans|lang_zh-Hant" name="lr">
<input type="hidden" value="0" name="c2coff">
<p align="center">
<img alt="Google" src="http://www.google.com/intl/en_us/images/logo.gif" />
</p>
<p align="center">
<input size="50" name="q">
<input type="submit" value="Search" name="sa">
</p>
</form>
<p align="center">
Koncord Private Search Engine Based on New Google Technology
</p>
</body>
</html>
http://www.google.com/coop/docs/cse/resultsxml.html
cx - Required. The cx parameter specifies a unique code that identifies a custom search engine. You must specify a Custom Search Engine using the cx parameter to retrieve search results from that CSE.
ie - Optional. The ie parameter sets the character encoding scheme that should be used to interpret the query string. The default ie value is latin1.
q - Optional. The q parameter specifies the search query entered by the user. Even though this parameter is optional, you must specify a value for at least one of the query parameters (as_epq, as_lq, as_oq, as_q, as_rq) to get search results.
sa - Required. It is indeed you submit the search query to Google search engine.
Today, we add three more parameters to show how the Simplified Chinese and Traditional Chinese can be interchangeable as search result.
hl - Optional. The hl parameter specifies the interface language (host language) of your user interface. To improve the performance and the quality of your search results, you are strongly encouraged to set this parameter explicitly. However, a successful search engine does not rely only on the parameters, but also settings of search engine itself. That is why you may also need your own account. “Site language”, is not only the site language, but also the search engine host language (the display language other than search result). Naturally, it should be set as English. “Your site encoding”, which is the searching language, should be GB18030 in this example.
lr - Optional. The lr (language restrict) parameter restricts search results to documents written in a particular language. Google WebSearch determines the language of a document by analyzing:
- the top-level domain (TLD) of the document's URL
- language meta tags within the document
- the primary language used in the body text of the document
c2coff - Optional. The c2coff parameter enables or disables the Simplified and Traditional Chinese Search feature. Please note, this parameter is only related to Chinese. For other language, no need to use this. The default value for this parameter is "0" (zero), meaning that the feature is enabled. Values for the c2coff parameter are: 1 is disabled, and 0 is enabled.
Following is codes with these two parameters. It means the search will be restricted to only websites wrote in three languages: US English, Simplified Chinese and Traditional Chinese.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en">
<head>
<title>
This is private search engine using Google facility
</title>
</head>
<body>
</br>
</br>
</br>
</br>
</br>
</br>
</br>
</br>
<form id="cse-search-box" action="http://www.google.com/cse">
<input type="hidden" value="partner-pub-3597878264183301:140hrt-vklq" name="cx">
<input type="hidden" value="GB18030" name="ie">
<input type="hidden" value="lang_en|lang_zh-Hans|lang_zh-Hant" name="lr">
<input type="hidden" value="0" name="c2coff">
<p align="center">
<img alt="Google" src="http://www.google.com/intl/en_us/images/logo.gif" />
</p>
<p align="center">
<input size="50" name="q">
<input type="submit" value="Search" name="sa">
</p>
</form>
<p align="center">
Koncord Private Search Engine Based on New Google Technology
</p>
</body>
</html>
http://www.google.com/coop/docs/cse/resultsxml.html
Create Your Own Search Engine
Following is the most basic HTML code to create the custom search engine based on Google’s technology. Actually, it just passes parameters to Google’s search engine. If you have your own AdSense account, replace it with yours. You can always change the words and contents as you wish. You may also wish to save it as home page for your browser.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en">
<head>
<title>
This is private search engine using Google facility
</title>
</head>
<body>
</br>
</br>
</br>
</br>
</br>
</br>
</br>
</br>
<form id="cse-search-box" action="http://www.google.com/cse">
<input type="hidden" value="partner-pub-3597878264183301:140hrt-vklq" name="cx">
<input type="hidden" value="ISO-8859-1" name="ie">
<p align="center">
<img alt="Google" src="http://www.google.com/intl/en_us/images/logo.gif" />
</p>
<p align="center">
<input size="50" name="q">
<input type="submit" value="Search" name="sa">
</p>
</form>
<p align="center">
Koncord Private Search Engine Based on New Google Technology
</p>
</body>
</html>
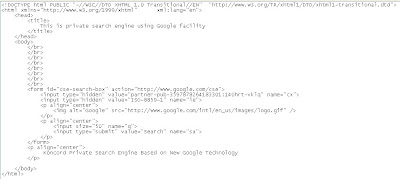
Following is the most essential part of the HTML. Save it as HTML format and replace “<“ with “<” since blogspot.com using HTML and it is not possible to publish .
<html>
<body>
<form id="cse-search-box" action="http://www.google.com/cse">
<input type="hidden" value="partner-pub-3597878264183301:140hrt-vklq" name="cx">
<input size="50" name="q">
<input type="submit" value="Search" name="sa">
</form>
</body>
</html>
That is what looks like:
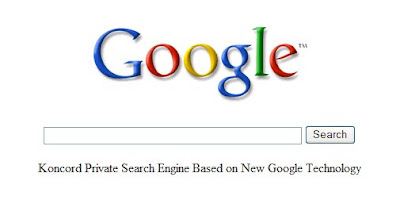
http://www.google.com/coop/docs/cse/resultsxml.html
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en">
<head>
<title>
This is private search engine using Google facility
</title>
</head>
<body>
</br>
</br>
</br>
</br>
</br>
</br>
</br>
</br>
<form id="cse-search-box" action="http://www.google.com/cse">
<input type="hidden" value="partner-pub-3597878264183301:140hrt-vklq" name="cx">
<input type="hidden" value="ISO-8859-1" name="ie">
<p align="center">
<img alt="Google" src="http://www.google.com/intl/en_us/images/logo.gif" />
</p>
<p align="center">
<input size="50" name="q">
<input type="submit" value="Search" name="sa">
</p>
</form>
<p align="center">
Koncord Private Search Engine Based on New Google Technology
</p>
</body>
</html>
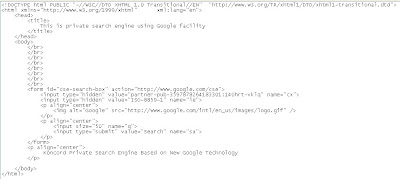
Following is the most essential part of the HTML. Save it as HTML format and replace “<“ with “<” since blogspot.com using HTML and it is not possible to publish .
<html>
<body>
<form id="cse-search-box" action="http://www.google.com/cse">
<input type="hidden" value="partner-pub-3597878264183301:140hrt-vklq" name="cx">
<input size="50" name="q">
<input type="submit" value="Search" name="sa">
</form>
</body>
</html>
That is what looks like:
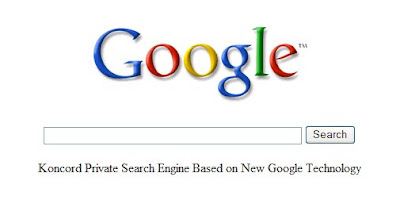
http://www.google.com/coop/docs/cse/resultsxml.html
Install Oracle Enterprise Linux on VMware Server
Atul Kumar has provided very clear procedures how to download and install VMware 2.0 and Oracle Enterprise Linux for Oracle database in http://onlineappsdba.com/index.php/2009/01/26/download-install-vmware-20-and-oracle-enterprise-linux-for-oracle-database/.
This article is served as an supplement to Atul Kumar's procedure.
1.Download and Unzip Oracle Enterprise Linux 5
Click http://koncordpartners.blogspot.com/2009/07/download-oracle-enterprise-linux.html to learn more about this. Please download Oracle Enterprise Linux 5 instead of JeSO. Please do only unzip and do NOT extract files out from ISO files. The VMware will read ISO files directly.
2.Download and Install VMware Server on Windows Machine
Click http://koncordpartners.blogspot.com/2009/07/installation-of-vmwares-vmware-server.html to learn more about this.
3.Configure Linux Machine on VMware Server
First, create a local folder/driver to store VM related files. This can be a folder in your C:\ Driver. In Atul Kumar's example, it is E:\ Driver. Then, copy your unzipped Linux files into this folder. The Linux files should be in iso format. Now, you can start Atul Kumar's procedure No.3.
After click “Use an ISO Image”, please point to the first ISO disc file, such as “Enterprise-R5-U3-Sever-i386-disc1.iso”.
4.Install Oracle Enterprise Linux 5 on VMware Server 2.0
Atul Kumar's procedure No.4 indeed is another article: http://onlineappsdba.com/index.php/2009/01/28/install-oracle-enterprise-linux-52-on-vmware-server-20/.
In VMware, click your VM in Inventory at left hand side, you will see Console appears on the middle pane. Click it, if you have not install Console, install it now.
After finish install iso disc 1, you will be prompted to change CDROM: Please insert Enterprise Linux disc 2 to continue. You need to:
a) Press Ctrl Alt. This enable you switch from Linux to Windows. Ctrl G will enable you switch from Windows to Linus.
b) Move mouse to the top of Linux installation window, click Devices.
c) You need to first disconnect the previous disc you have done, by click Disconnect...(the name of your previous Linux disc), next to CD/DVD Drive 1.
d) A pop-up window will ask you to confirm this action. Click Yes.
e) Move mouse to the top of Linux installation window, click Devices.
f) Click CD/DVD Drive 1.
g) Click Connect to Disk Image File (iso).
h) Find “Enterprise-R5-U3-Sever-i386-disc2.iso”.
Do the same for rest discs.
5.Install 10g Database or Oracle Apps 11i/R12 on Oracle Enterprise Linux
Sure, it is not limited to 10g Database or Oracle Apps 11i/R12 only.
http://onlineappsdba.com/index.php/2009/01/26/download-install-vmware-20-and-oracle-enterprise-linux-for-oracle-database/
http://onlineappsdba.com/index.php/2009/01/28/install-oracle-enterprise-linux-52-on-vmware-server-20/
http://onlineappsdba.com/index.php/2007/03/04/install-oracle-enterprise-linux-on-vmware/
This article is served as an supplement to Atul Kumar's procedure.
1.Download and Unzip Oracle Enterprise Linux 5
Click http://koncordpartners.blogspot.com/2009/07/download-oracle-enterprise-linux.html to learn more about this. Please download Oracle Enterprise Linux 5 instead of JeSO. Please do only unzip and do NOT extract files out from ISO files. The VMware will read ISO files directly.
2.Download and Install VMware Server on Windows Machine
Click http://koncordpartners.blogspot.com/2009/07/installation-of-vmwares-vmware-server.html to learn more about this.
3.Configure Linux Machine on VMware Server
First, create a local folder/driver to store VM related files. This can be a folder in your C:\ Driver. In Atul Kumar's example, it is E:\ Driver. Then, copy your unzipped Linux files into this folder. The Linux files should be in iso format. Now, you can start Atul Kumar's procedure No.3.
After click “Use an ISO Image”, please point to the first ISO disc file, such as “Enterprise-R5-U3-Sever-i386-disc1.iso”.
4.Install Oracle Enterprise Linux 5 on VMware Server 2.0
Atul Kumar's procedure No.4 indeed is another article: http://onlineappsdba.com/index.php/2009/01/28/install-oracle-enterprise-linux-52-on-vmware-server-20/.
In VMware, click your VM in Inventory at left hand side, you will see Console appears on the middle pane. Click it, if you have not install Console, install it now.
After finish install iso disc 1, you will be prompted to change CDROM: Please insert Enterprise Linux disc 2 to continue. You need to:
a) Press Ctrl Alt. This enable you switch from Linux to Windows. Ctrl G will enable you switch from Windows to Linus.
b) Move mouse to the top of Linux installation window, click Devices.
c) You need to first disconnect the previous disc you have done, by click Disconnect...(the name of your previous Linux disc), next to CD/DVD Drive 1.
d) A pop-up window will ask you to confirm this action. Click Yes.
e) Move mouse to the top of Linux installation window, click Devices.
f) Click CD/DVD Drive 1.
g) Click Connect to Disk Image File (iso).
h) Find “Enterprise-R5-U3-Sever-i386-disc2.iso”.
Do the same for rest discs.
5.Install 10g Database or Oracle Apps 11i/R12 on Oracle Enterprise Linux
Sure, it is not limited to 10g Database or Oracle Apps 11i/R12 only.
http://onlineappsdba.com/index.php/2009/01/26/download-install-vmware-20-and-oracle-enterprise-linux-for-oracle-database/
http://onlineappsdba.com/index.php/2009/01/28/install-oracle-enterprise-linux-52-on-vmware-server-20/
http://onlineappsdba.com/index.php/2007/03/04/install-oracle-enterprise-linux-on-vmware/
Installation of VMware's VMware Server
First, what is it? VMware Server is a software enable you to create a multiple virtual machines within your existing operating system. It is not a virtual machine itself.
VMware Server is a Virtual Private Server (Server Virtualization). A VPS, also referred to as Virtual Dedicated Server (VDS), is a method of partitioning a physical server computer into multiple servers such that each has the appearance and capabilities of running on its own dedicated machine. Each virtual server can run its own full-fledged operating system, and each server can be independently rebooted. The physical server boots normally. It then runs a program that boots each virtual server within a virtualization environment. The virtual servers have no direct access to hardware and are usually booted from a disk image. A disk image is a single file or storage device containing the complete contents and structure representing a data storage medium or device, such as a hard drive, CD, or DVD. Some disk imaging utilities omit unused file space from source media, or compress the disk they represent to reduce storage requirements, though these are typically referred to as archive files, as they are not literally disk images. The most common format of disk image is ISO.
Install and run VMware Server as an application on top of a host Windows or Linux operating system. A thin virtualization layer partitions the physical server so you can run multiple virtual machines simultaneously on a single server. Computing resources of the physical server are treated as a uniform pool of resources that can be allocated to virtual machines in a controlled manner.
VMware Server isolates each virtual machine from its host and other virtual machines, leaving it unaffected if another virtual machine crashes. Your data does not leak across virtual machines and your applications can only communicate over configured network connections. VMware Server encapsulates a virtual machine environment as a set of files, which are easy to back-up, move and copy.
According to Vmware, VMware Server is typically used in the following scenarios:
■Assessing virtualization for the 1st time
■Evaluating software using virtual machines
■Test and development of software and IT environments
Following matrix can help to determine if VMware Server is suitable:
.jpg)
https://www.vmware.com/freedownload/login.php?product=server20 is the site for download. A free registration is needed. Go to your email account to activiate before download. In “Binaries”, select the option in according with the current operating system. During installation process, you may also prompt to enter serial number, which can be find in the same email.
A icon of VMware Server Home Page will be created. However, when click this icon, someone might be found https://[localhost, usually it is your computer name; no square brackets]:8333/ui/# could not be acceessed. In this case, you would need to try again and set allow intranet.
Some may find following message:
There is a problem with this website's security certificate.
The security certificate presented by this website was not issued by a trusted certificate authority.
Security certificate problems may indicate an attempt to fool you or intercept any data you send to the server.
We recommend that you close this webpage and do not continue to this website.
- Click here to close this webpage.
- Continue to this website (not recommended).
- More information.
Please click “Continue to this website (not recommended).”
Then, when you try to access VMware Server, you may get an error message saying "Access Denied" or ask you to enter the Login Name and Password in a pop-up window of VMware Infrastructure Web Access. Please use your Window's Login Name and Password. Remember, you must have Administrator previlege in your PC.
By now, you should have no problem to download, install and login the VMware Server.
Rating? Five Stars from us.
http://www.vmware.com/products/server/overview.html
http://en.wikipedia.org/wiki/Server_virtualization
http://en.wikipedia.org/wiki/Disk_image
http://www.electrictoolbox.com/access-denied-vmware-infrastructure-web-access/
VMware Server is a Virtual Private Server (Server Virtualization). A VPS, also referred to as Virtual Dedicated Server (VDS), is a method of partitioning a physical server computer into multiple servers such that each has the appearance and capabilities of running on its own dedicated machine. Each virtual server can run its own full-fledged operating system, and each server can be independently rebooted. The physical server boots normally. It then runs a program that boots each virtual server within a virtualization environment. The virtual servers have no direct access to hardware and are usually booted from a disk image. A disk image is a single file or storage device containing the complete contents and structure representing a data storage medium or device, such as a hard drive, CD, or DVD. Some disk imaging utilities omit unused file space from source media, or compress the disk they represent to reduce storage requirements, though these are typically referred to as archive files, as they are not literally disk images. The most common format of disk image is ISO.
Install and run VMware Server as an application on top of a host Windows or Linux operating system. A thin virtualization layer partitions the physical server so you can run multiple virtual machines simultaneously on a single server. Computing resources of the physical server are treated as a uniform pool of resources that can be allocated to virtual machines in a controlled manner.
VMware Server isolates each virtual machine from its host and other virtual machines, leaving it unaffected if another virtual machine crashes. Your data does not leak across virtual machines and your applications can only communicate over configured network connections. VMware Server encapsulates a virtual machine environment as a set of files, which are easy to back-up, move and copy.
According to Vmware, VMware Server is typically used in the following scenarios:
■Assessing virtualization for the 1st time
■Evaluating software using virtual machines
■Test and development of software and IT environments
Following matrix can help to determine if VMware Server is suitable:
.jpg)
https://www.vmware.com/freedownload/login.php?product=server20 is the site for download. A free registration is needed. Go to your email account to activiate before download. In “Binaries”, select the option in according with the current operating system. During installation process, you may also prompt to enter serial number, which can be find in the same email.
A icon of VMware Server Home Page will be created. However, when click this icon, someone might be found https://[localhost, usually it is your computer name; no square brackets]:8333/ui/# could not be acceessed. In this case, you would need to try again and set allow intranet.
Some may find following message:
There is a problem with this website's security certificate.
The security certificate presented by this website was not issued by a trusted certificate authority.
Security certificate problems may indicate an attempt to fool you or intercept any data you send to the server.
We recommend that you close this webpage and do not continue to this website.
- Click here to close this webpage.
- Continue to this website (not recommended).
- More information.
Please click “Continue to this website (not recommended).”
Then, when you try to access VMware Server, you may get an error message saying "Access Denied" or ask you to enter the Login Name and Password in a pop-up window of VMware Infrastructure Web Access. Please use your Window's Login Name and Password. Remember, you must have Administrator previlege in your PC.
By now, you should have no problem to download, install and login the VMware Server.
Rating? Five Stars from us.
http://www.vmware.com/products/server/overview.html
http://en.wikipedia.org/wiki/Server_virtualization
http://en.wikipedia.org/wiki/Disk_image
http://www.electrictoolbox.com/access-denied-vmware-infrastructure-web-access/
Detect a Leap Year with PL/SQL
We have seen some very complicated ways to detect leap year. Follow functions are probably the most elegant method we can find:
function DATE_LEAP_YEAR_IS
--------------------------------------------------------------------------------
-- This function is to return if a given year is leap year or not.
-- This is 1 of 2 overloaded functions.
-- Input: Parameter 1: The given year in number.
-- Output: Return: The result in single varchar2, 'Y' is leap year, 'N' is not.
-- Authers: Ken Stevens, Lee Howe, Jane Stone & Howard Stone
--------------------------------------------------------------------------------
        (      nYr in number
        )      return varchar2
as v_day varchar2(2);
begin
        v_day := to_char(last_day(to_date(to_char(nYr)||'/02/01', 'YYYY/MM/DD')), 'DD');
        if v_day = '29' -- if v_day = 29 then it must be a leap year, return TRUE.
        then return 'Y';
        else return 'N';
        end if;
end DATE_LEAP_YEAR_IS;
function DATE_LEAP_YEAR_IS
--------------------------------------------------------------------------------
-- This function is to return if a given year is leap year or not.
-- This is 1 of 2 overloaded functions.
-- Input: Parameter 1: The given year in date.
-- Output: Return: The result in single varchar2, 'Y' is leap year, 'N' is not.
-- Authers: Ken Stevens, Lee Howe, Jane Stone & Howard Stone
--------------------------------------------------------------------------------
        (      dDate in date
        )      return      varchar2
as v_day varchar2(2);
begin
        v_day := to_char(last_day(to_date(to_char(extract(year from dDate))||'/02/01', 'YYYY/MM/DD')), 'DD');
        if v_day = '29' -- if v_day = 29 then it must be a leap year, return TRUE.
        then return 'Y';
        else return 'N';
        end if;
end DATE_LEAP_YEAR_IS;
Following is Don Burleson’s scripts:
create or replace function IS_LEAP_YEAR (nYr in number) return boolean is
v_day varchar2(2);
begin
    select to_char(last_day(to_date( '01-FEB-'|| to_char(nYr), 'DD-MON-YYYY')), 'DD') into v_day from dual;
    if v_day = '29' then -- if v_day = 29 then it must be a leap year, return TRUE
        return TRUE;
    else
        return FALSE;    -- otherwise year is not a leap year, return false
    end if;
end;
http://www.dba-oracle.com/t_detect_leap_year_function.htm
http://www.dba-oracle.com/oracle_news/2005_8_23_function_detect_leap_year.htm
function DATE_LEAP_YEAR_IS
--------------------------------------------------------------------------------
-- This function is to return if a given year is leap year or not.
-- This is 1 of 2 overloaded functions.
-- Input: Parameter 1: The given year in number.
-- Output: Return: The result in single varchar2, 'Y' is leap year, 'N' is not.
-- Authers: Ken Stevens, Lee Howe, Jane Stone & Howard Stone
--------------------------------------------------------------------------------
        (      nYr in number
        )      return varchar2
as v_day varchar2(2);
begin
        v_day := to_char(last_day(to_date(to_char(nYr)||'/02/01', 'YYYY/MM/DD')), 'DD');
        if v_day = '29' -- if v_day = 29 then it must be a leap year, return TRUE.
        then return 'Y';
        else return 'N';
        end if;
end DATE_LEAP_YEAR_IS;
function DATE_LEAP_YEAR_IS
--------------------------------------------------------------------------------
-- This function is to return if a given year is leap year or not.
-- This is 1 of 2 overloaded functions.
-- Input: Parameter 1: The given year in date.
-- Output: Return: The result in single varchar2, 'Y' is leap year, 'N' is not.
-- Authers: Ken Stevens, Lee Howe, Jane Stone & Howard Stone
--------------------------------------------------------------------------------
        (      dDate in date
        )      return      varchar2
as v_day varchar2(2);
begin
        v_day := to_char(last_day(to_date(to_char(extract(year from dDate))||'/02/01', 'YYYY/MM/DD')), 'DD');
        if v_day = '29' -- if v_day = 29 then it must be a leap year, return TRUE.
        then return 'Y';
        else return 'N';
        end if;
end DATE_LEAP_YEAR_IS;
Following is Don Burleson’s scripts:
create or replace function IS_LEAP_YEAR (nYr in number) return boolean is
v_day varchar2(2);
begin
    select to_char(last_day(to_date( '01-FEB-'|| to_char(nYr), 'DD-MON-YYYY')), 'DD') into v_day from dual;
    if v_day = '29' then -- if v_day = 29 then it must be a leap year, return TRUE
        return TRUE;
    else
        return FALSE;    -- otherwise year is not a leap year, return false
    end if;
end;
http://www.dba-oracle.com/t_detect_leap_year_function.htm
http://www.dba-oracle.com/oracle_news/2005_8_23_function_detect_leap_year.htm
Download Oracle VM Template
Oracle VM and VMware are same things, because they all provide a virtual machine. And no, they are different. Oracle VM is based on the open-source Xen hypervisor technology, supports both Windows and Linux guests and includes an integrated Web browser based management console. Oracle VM features fully tested and certified Oracle Applications stack in an enterprise virtualization environment. However, that's not to say Oracle products won't run on the other virtualization servers. If the purpose to install the VM is not only for Oracle products, one should consider VMware or VirtualBox.
When go to http://edelivery.oracle.com/EPD/GetUserInfo/get_form?caller=LinuxWelcome, there are two options to chose, Oracle VM and Oracle VM Templates. The difference between them is the Oracle VM Templates are “fully configured software stack by offering pre-installed and pre-configured software images. Use of Oracle VM Templates eliminates the installation and configuration costs, and reduces the ongoing maintenance costs helping organizations achieve faster time to market and lower cost of operations. Oracle VM Templates of many key Oracle products are available for download, including Oracle Database, Enterprise Linux, Fusion Middleware, and many more.” At least these are Oracle’s objectives, if one knows how to download and how to install. One must remember Oracle VM Template could not work standalone. It must work with the Oracle VM Maanger.
Following options might be most selected:
- Oracle VM Templates for Oracle Database Media Pack for x86 (32 bit) 2.2.0.0.0 B51904-02
- Oracle VM Templates for Oracle Enterprise Linux 5 Media Pack for x86 (32 bit) 2.2.0.0.0 B52024-03
There are a few abbreviations worthwhile to know:
HVM – stands for Hardware Virtual Machine, and is usually used in reference to AMD’s and Intel’s Virtualization technology included in their new processors (Intel VT and AMD Pacifica).
PV – stands for paravirtualization, a virtualization technique that presents a software interface to virtual machines that is similar but not identical to that of the underlying hardware.
http://en.wikipedia.org/wiki/Virtual_machine
http://en.wikipedia.org/wiki/Oracle_VM
http://www.oracle.com/technology/products/vm/templates/index.html
http://www.crucialp.com/blog/2008/04/21/what-is-hvm/
When go to http://edelivery.oracle.com/EPD/GetUserInfo/get_form?caller=LinuxWelcome, there are two options to chose, Oracle VM and Oracle VM Templates. The difference between them is the Oracle VM Templates are “fully configured software stack by offering pre-installed and pre-configured software images. Use of Oracle VM Templates eliminates the installation and configuration costs, and reduces the ongoing maintenance costs helping organizations achieve faster time to market and lower cost of operations. Oracle VM Templates of many key Oracle products are available for download, including Oracle Database, Enterprise Linux, Fusion Middleware, and many more.” At least these are Oracle’s objectives, if one knows how to download and how to install. One must remember Oracle VM Template could not work standalone. It must work with the Oracle VM Maanger.
Following options might be most selected:
- Oracle VM Templates for Oracle Database Media Pack for x86 (32 bit) 2.2.0.0.0 B51904-02
- Oracle VM Templates for Oracle Enterprise Linux 5 Media Pack for x86 (32 bit) 2.2.0.0.0 B52024-03
There are a few abbreviations worthwhile to know:
HVM – stands for Hardware Virtual Machine, and is usually used in reference to AMD’s and Intel’s Virtualization technology included in their new processors (Intel VT and AMD Pacifica).
PV – stands for paravirtualization, a virtualization technique that presents a software interface to virtual machines that is similar but not identical to that of the underlying hardware.
http://en.wikipedia.org/wiki/Virtual_machine
http://en.wikipedia.org/wiki/Oracle_VM
http://www.oracle.com/technology/products/vm/templates/index.html
http://www.crucialp.com/blog/2008/04/21/what-is-hvm/
Download Oracle Enterprise Linux
So, you have problem to install an Oracle product in your PC. That is right, many operating systems are not certified by Oracle products, which means you are unable to install these Oracle products on this OS, or they won't work properly after installation. The Oracle products are indeed written to run on UNIX or Linux systems. So you decision to install Oracle Enterprise Linux is a right approach.
To download Oracle Enterprise Linux,
1. You can go directly to following URL: http://edelivery.oracle.com/EPD/GetUserInfo/get_form?caller=LinuxWelcome.
2. Fill your details. Many Oracle products are free to download. Same is true for Oracle Enterprise Linux.
3. In next screen, pick Enterprise Linux in “Select a Product Pack”. In “Platform”, pick up what you want. You have three options: IA64, x86 32 bit and x86 64bit. IA64 is the formal name of Itanium, a family of 64-bit Intel microprocessors that implement the Intel Itanium architecture. If you do not know what your computer is, check here: http://support.microsoft.com/kb/827218.
4. Don’t know what version to download? Here are comparisons: http://distrowatch.com/table.php?distribution=oracle. If the purpose is to install Oracle Enterprise Linux based on Oracle VM Templates, the best option would be Oracle Enterprise Linux JeOS for Building Oracle VM templates Media Pack v5 for x86 (32 bit).
http://www.oracle.com/technology/software/products/virtualization/vm_jeos.html
To download Oracle Enterprise Linux,
1. You can go directly to following URL: http://edelivery.oracle.com/EPD/GetUserInfo/get_form?caller=LinuxWelcome.
2. Fill your details. Many Oracle products are free to download. Same is true for Oracle Enterprise Linux.
3. In next screen, pick Enterprise Linux in “Select a Product Pack”. In “Platform”, pick up what you want. You have three options: IA64, x86 32 bit and x86 64bit. IA64 is the formal name of Itanium, a family of 64-bit Intel microprocessors that implement the Intel Itanium architecture. If you do not know what your computer is, check here: http://support.microsoft.com/kb/827218.
4. Don’t know what version to download? Here are comparisons: http://distrowatch.com/table.php?distribution=oracle. If the purpose is to install Oracle Enterprise Linux based on Oracle VM Templates, the best option would be Oracle Enterprise Linux JeOS for Building Oracle VM templates Media Pack v5 for x86 (32 bit).
http://www.oracle.com/technology/software/products/virtualization/vm_jeos.html
Date Format
People in United States use date format of mm/dd/yyyy. British, Australia and New Zealand use dd/mm/yyyy. Format of yyyy-mm-dd is an ISO 8601 international standard, and is favored by European countries and all Chinese spoken countries. Canada is THE country currently using all of these three formats. Format of yyyy-mm-dd is official format for Canada. Because they are a Commonwealth member country, they also use British format. And because they are heavily influenced by United States, in day-to-day life Canadians use American format most.
It is for sure IT industry should use yyyy-mm-dd format, not because it is an ISO 8601 standard, but also it is only meaningful format when you conduct textual sorting. For instance, when you use date as part of file name, it can be directly sorted by Windows Explorer.
In Microsoft Excel, if yyyy-mm-dd format is needed, please go to Format Cells, chose Locale as Chinese (Hong Kong S.A.R). It can be found in Type.
Sunday is traditionally regarded as the first day of the week. Different industries may have their own standard. However, the International Organization for Standardization (ISO) specifies that the week begins with Monday, which is also the standard for IT industry. So, day 1 in a week is Monday. The week ends at 23:59:59 of Sunday. Beware, Microsoft Excel's function weekday() does use Sunday as day 1 in a week. In addition, in Microsoft SQL Server DATEPART(WEEKDAY, dateToCheck) does also generate 2 for Monday.
Look at following three times:
Time 1: 2000-01-01 11:59:59 AM
Time 2: 2000-01-01 12:00:00
Time 3: 2000-01-01 12:00:01 PM
How many seconds between Time 1 and Time 2 above? How many seconds between Time 2 and Time 3? Are you sure? Would you think again, please? We human being just would like to confuse ourselves. Then look at these:
Time a: 2000-01-01 11:59:59 PM
Time b: 2000-01-01 0:00:00
Time c: 2000-01-01 0:00:01 AM
Time d: 2000-01-01 0:59:59 AM
Time e: 2000-01-01 1:00:00 PM
Time e: 2000-01-01 1:00:01 AM
So, if it is possible, please use military time (24 hour time system) instead of 12 hour time system.
Look at following three times:
Time 4: 2000-01-01 00:00:00
Time 5: 2000-01-01 24:00:00
Time 6: 2000-01-02 00:00:00
Are they at same time? Indeed, the format of Time 5 would never exist in any official document, except in some railway time tables, where 24:00:00 used only to describe the arrival time. Time 4 and Time 6 are often confused, though there is 24 hours difference. However, for the purpose of reporting period ending time, it is suggested to use format either as 1999-12-31 24:00:00 or 1999-12-31 23:59:59, but not 2000-01-01 00:00:00. This is particularly important for week report, because the reader could not tell from the glance at the date. For instance, 2010-04-12 00:00:00, how can you directly tell it is one second from 2010-04-11 00:00:01 or 2010-04-12 23:59:59? No, both are incorrect. The correct answer is it is one second from 2010-04-12 00:00:01 or 2010-04-11 23:59:59.
When the first week starts in a year? ISO 8601 provides the first week in a year starts from a Monday of a week with includes the first Thursday in that year. Therefore, the first week must include the January 4th. This approach does indeed emphasis the majority of the days in first week are in new year. This approach can also be used to determine the first week of a fiscal year as well as the first week of a month.
http://en.wikipedia.org/wiki/Date_and_time_notation_by_country
http://en.wikipedia.org/wiki/Calendar_date
http://en.wikipedia.org/wiki/ISO_8601
http://24hourtime.info/the-24-hour-time-system/
http://www.cl.cam.ac.uk/~mgk25/iso-time.html
http://en.wikipedia.org/wiki/12-hour_clock
It is for sure IT industry should use yyyy-mm-dd format, not because it is an ISO 8601 standard, but also it is only meaningful format when you conduct textual sorting. For instance, when you use date as part of file name, it can be directly sorted by Windows Explorer.
In Microsoft Excel, if yyyy-mm-dd format is needed, please go to Format Cells, chose Locale as Chinese (Hong Kong S.A.R). It can be found in Type.
Sunday is traditionally regarded as the first day of the week. Different industries may have their own standard. However, the International Organization for Standardization (ISO) specifies that the week begins with Monday, which is also the standard for IT industry. So, day 1 in a week is Monday. The week ends at 23:59:59 of Sunday. Beware, Microsoft Excel's function weekday() does use Sunday as day 1 in a week. In addition, in Microsoft SQL Server DATEPART(WEEKDAY, dateToCheck) does also generate 2 for Monday.
Look at following three times:
Time 1: 2000-01-01 11:59:59 AM
Time 2: 2000-01-01 12:00:00
Time 3: 2000-01-01 12:00:01 PM
How many seconds between Time 1 and Time 2 above? How many seconds between Time 2 and Time 3? Are you sure? Would you think again, please? We human being just would like to confuse ourselves. Then look at these:
Time a: 2000-01-01 11:59:59 PM
Time b: 2000-01-01 0:00:00
Time c: 2000-01-01 0:00:01 AM
Time d: 2000-01-01 0:59:59 AM
Time e: 2000-01-01 1:00:00 PM
Time e: 2000-01-01 1:00:01 AM
So, if it is possible, please use military time (24 hour time system) instead of 12 hour time system.
Look at following three times:
Time 4: 2000-01-01 00:00:00
Time 5: 2000-01-01 24:00:00
Time 6: 2000-01-02 00:00:00
Are they at same time? Indeed, the format of Time 5 would never exist in any official document, except in some railway time tables, where 24:00:00 used only to describe the arrival time. Time 4 and Time 6 are often confused, though there is 24 hours difference. However, for the purpose of reporting period ending time, it is suggested to use format either as 1999-12-31 24:00:00 or 1999-12-31 23:59:59, but not 2000-01-01 00:00:00. This is particularly important for week report, because the reader could not tell from the glance at the date. For instance, 2010-04-12 00:00:00, how can you directly tell it is one second from 2010-04-11 00:00:01 or 2010-04-12 23:59:59? No, both are incorrect. The correct answer is it is one second from 2010-04-12 00:00:01 or 2010-04-11 23:59:59.
When the first week starts in a year? ISO 8601 provides the first week in a year starts from a Monday of a week with includes the first Thursday in that year. Therefore, the first week must include the January 4th. This approach does indeed emphasis the majority of the days in first week are in new year. This approach can also be used to determine the first week of a fiscal year as well as the first week of a month.
http://en.wikipedia.org/wiki/Date_and_time_notation_by_country
http://en.wikipedia.org/wiki/Calendar_date
http://en.wikipedia.org/wiki/ISO_8601
http://24hourtime.info/the-24-hour-time-system/
http://www.cl.cam.ac.uk/~mgk25/iso-time.html
http://en.wikipedia.org/wiki/12-hour_clock
Subscribe to:
Posts (Atom)
Labels
- :: (1)
- ? (1)
- .bat (1)
- .css (1)
- .getElementById (1)
- .htaccess (2)
- .html (1)
- .iso (3)
- .js (2)
- .js.php (2)
- .length (1)
- .parent (1)
- .php (1)
- .replace() (3)
- .replace(RegExp) (2)
- .search() (2)
- .SendMail (1)
- .sql (1)
- .style.height (1)
- .write (1)
- 'N' (1)
- 'null' (1)
- 'title' (1)
- 'undefined' (2)
- "Canvas" (1)
- "top()" (1)
- ( (1)
- () (1)
- (a) (1)
- (a)count() (1)
- [ (1)
- [...] (1)
- [0] (1)
- [rsInvalidDataSetName] The table ‘table1’ refers to an invalid DataSetName (1)
- { (1)
- * (1)
- \ (1)
- \n (2)
- \t (1)
- % (2)
- %...% (1)
- ^ (1)
- + (1)
- | (1)
- $ (1)
- $end (1)
- $this- (1)
- 0 (1)
- 1 OR -1 (1)
- 1280x1024 (1)
- 1680x1050 (1)
- 1920x1200 (1)
- 1σ (1)
- 2560x1600 (1)
- 32-bit (2)
- 34.1% (1)
- 3rd Normal Form (1)
- 64-bit (2)
- 7680x4800 (1)
- a (2)
- Access (1)
- Across Different Rows (1)
- Across HTML Pages (1)
- Action Query (1)
- Active (1)
- ActiveWorkbook (1)
- ADD COLUMN (1)
- Address (1)
- ADDRESS() (1)
- AdSense (1)
- Advanced Editing Toolbar (1)
- Aggragate function (1)
- AJAX (2)
- Algorithm (1)
- ALTER TABLE (1)
- Analytic Functions (1)
- Anchor (1)
- Annualized Projection (1)
- Anonymous Function (1)
- Another Table (1)
- ANSI SQL (1)
- Append Array Into Another Array (1)
- ArcCatalog (1)
- ArcEditor (2)
- ArcGIS (1)
- ArcMap (1)
- Arithmetic Mean (1)
- Array (6)
- Array Data Type (1)
- Array Slice (1)
- Array Type (1)
- array_merge() (1)
- array_push() (1)
- Artificial Intelligence (1)
- ASCII (1)
- ASCII Key Code (1)
- ASPX (1)
- Assembly (1)
- Associative Array (3)
- Attribute (2)
- Atul Kumar (1)
- Auto-Number (1)
- AUTOINCREMENT (1)
- Automatic (1)
- Automatic Login (1)
- Automatically Added Element (1)
- Automation (3)
- availHeight (1)
- AVG() (1)
- Aviation (1)
- Background Image (1)
- Batch File (1)
- bcc (1)
- Best Length (1)
- BI (2)
- Big5 (1)
- Bind Variable (5)
- blog.dwnews.com (1)
- Bookmarks (1)
- Boolean (1)
- Bracket (4)
- Bracket () (1)
- Browser (1)
- Bug (2)
- Bulk (1)
- Bulk Collect (1)
- Business Intelligence (2)
- Button (1)
- C# (1)
- c2coff (1)
- Calculation (1)
- Calendar Control (1)
- Caps Lock (1)
- CASE (5)
- CAST (1)
- cc (1)
- CD/DVD (1)
- CDO (1)
- CEIL (1)
- Cell (3)
- Charset (1)
- Checkbox (1)
- Chinese Characters (2)
- Chute Time (1)
- Circle (1)
- Class (2)
- Class Selector (1)
- Clean Code (1)
- Clean Computer (1)
- clientHeight (1)
- Clipboard (1)
- Closing Identifier (1)
- Closures (1)
- Code Editor (1)
- Code Cleaning (3)
- Code Cleanser (1)
- Code Compress (1)
- Code Compression (1)
- Code Compressor (1)
- Code Conventions (4)
- Code Optimization (1)
- Code Optimizer (1)
- Collection (1)
- Color Code in HTML (1)
- Column Alias (1)
- Column Name (3)
- Comma (1)
- Comments (2)
- Compact and Repair (1)
- Comparison (1)
- Comparison of IF Statement (1)
- Computer Science (1)
- Concatenation (1)
- Condition Set (1)
- Conditions (1)
- contentWindow (1)
- Convert (1)
- Convert String To Reference (1)
- CONVERT() (4)
- Coordinates Parse (1)
- Copy (3)
- count() (1)
- Create (1)
- Create Table (1)
- CREATE VIEW (1)
- Crimson Editor (1)
- Cross Join (1)
- Cross Windows (1)
- Crystal Reports (2)
- Crystal X (1)
- Crystal XI (1)
- CSS (4)
- Ctri+Shit+Enter (1)
- cx (1)
- Data Connection Wizard (1)
- Data Layout (1)
- Data Layout in Report (1)
- Data Type (2)
- Data Warehouse (1)
- Database (3)
- Dataset (2)
- DataSetName (1)
- Datatype (1)
- Date Format (2)
- DATEADD() (1)
- DATEDIFF() (1)
- DATEPART() (1)
- Dates Inclusive (1)
- Days in Month (1)
- DBA (1)
- Debug (1)
- Decimal Place (2)
- Decimal Point (2)
- DECIMAL() (1)
- DECODE (1)
- Default Database (1)
- Delegate (1)
- DELETE (3)
- Deleted Records (1)
- Delimited By Comma (1)
- Denormalized Data Structure (1)
- Deprecation (1)
- Description (1)
- DHTML (8)
- Dialogue Window (1)
- Different Servers (1)
- DISABLE CONSTRAINT (1)
- Disk Image (1)
- Disk Space (1)
- Disorderly Sorting Criterion (1)
- DISTINCT (1)
- Distributed Computing (1)
- DIV (2)
- DO (1)
- document.body.appendChild() (1)
- document.body.clientHeight Does Not Work (1)
- document.body.clientWidth/Height (1)
- document.body.offsetWidth/Height (1)
- document.createElement() (1)
- document.documentElement.clientWidth/Height (1)
- document.getElementById().innerHTML (2)
- document.getElementById().value (1)
- document.write() (3)
- Does Not Calculate (1)
- DOM (1)
- domain (1)
- Don Burleson (1)
- Double Quote (1)
- Drag and Drop (1)
- Draw Circle (1)
- DROP (1)
- Drop Down List (1)
- DSN (1)
- Dump Tests (1)
- Dynamic (2)
- Dynamic Codes (2)
- Dynamic Column Name (1)
- Dynamic Column Numbers (2)
- Dynamic Columns (1)
- Dynamic Dimension (1)
- Dynamic HTML (7)
- Dynamic Query (3)
- Dynamic SQL (2)
- Dynamic Table Name (1)
- Element (1)
- Embed (2)
- Empty String (1)
- empty() (1)
- ENABLE CONSTRAINT (1)
- Enable PHP (3)
- Encapsulation (2)
- End Bracket (2)
- End Tag (2)
- Enforce Width (1)
- Equivalent of window.innerWidth/Height (1)
- Error (2)
- Error Console (1)
- Error Massage (1)
- Error Message (10)
- Error message: Operation must use an updateable query (1)
- Error Number 2950 (1)
- Error: Function expected (1)
- Error: Invalid argument (1)
- Error: is not a function (1)
- Escape (1)
- Escape Sequence (2)
- eval() (1)
- Event (2)
- Examination (1)
- Exams (1)
- Excel (6)
- Excel 2003 (1)
- Excel 2007 (1)
- Excel Functions (1)
- EXEC (3)
- EXEC sp_executesql (1)
- EXEC() (1)
- EXECUTE (2)
- EXECUTE() (1)
- Existing (1)
- Existing Table (1)
- Explain Plan (1)
- explode() (1)
- External Data (1)
- FALSE (1)
- Fast (1)
- Fatal error: Call to undefined function... (1)
- Favorites (1)
- FileFormat (1)
- Firefox (3)
- First Day in Week (1)
- First Element (1)
- First Week in Month (1)
- First Week in Year (1)
- Fiscal Year (1)
- Flag (1)
- Float (1)
- FLOOR (1)
- for...in (1)
- Force Download (1)
- Force Update (1)
- Forecast (1)
- Form (6)
- Format (2)
- Format Cells (1)
- Formula (2)
- Formula Shown Up (1)
- Friday (1)
- Function (2)
- Function Declaration (2)
- Function Literal (4)
- Function Object (1)
- Function Passing (2)
- Function Pointer (3)
- Function Reference (3)
- GB (1)
- GB18030 (2)
- GB2312 (1)
- GB2312-80 (1)
- General (1)
- Geodata (2)
- getElementId() (1)
- GIS (3)
- Global Temprary Table (1)
- Google (4)
- Google Maps (1)
- GROUP BY (3)
- GTT (1)
- Handwriting (1)
- Hardware Engineering (1)
- header() (1)
- Heredoc (1)
- Hexadecimal (1)
- Hierarchy (1)
- Historic Data (1)
- hl (1)
- Homepage (2)
- Horizontal (1)
- Hour (1)
- Hover (1)
- Howard Stone (1)
- href= (1)
- HTML (20)
- HTML Color Code (1)
- HTML Loading Sequence (1)
- HTML Shows Nothing (1)
- HTML Table (1)
- http (1)
- HVM (1)
- IA64 (1)
- IDE (1)
- Identifier (1)
- Identifier URL (1)
- Identify (1)
- ie (6)
- IE 8 (1)
- IE Bug (2)
- IF (1)
- IF ELSE (1)
- IF ELSE Statement (2)
- IF Statement (1)
- if() (1)
- iFrame (3)
- iFrame Height (1)
- IIF (1)
- IIF() (1)
- Image (1)
- Import and Export Data (32-bit) (1)
- Importing Identifier (1)
- IN (1)
- Include (1)
- Indent (1)
- Indentation (3)
- Index (2)
- Indexed Array (2)
- INDIRECT() (1)
- Information Management (1)
- Information Science (1)
- Information Technology (1)
- Inheritance (1)
- INNER JOIN (2)
- Inner Query (2)
- innerHeight (1)
- Input (1)
- Input Item (1)
- Insert (2)
- Installer Structure (1)
- Instantiation (1)
- INT (1)
- Integer (1)
- Interface (2)
- Internet Explorer (4)
- Internet Explorer 8 (1)
- Interquartile Mean (2)
- Intersection (1)
- Invalid Argument (1)
- IQM (3)
- is not a function (1)
- IS NULL (1)
- Is Number (1)
- Is Numeric (1)
- is_float() (1)
- is_int() (2)
- is_null() (1)
- is_numeric() (3)
- Is_numeric() 0 (1)
- is_string() (1)
- isNumber (1)
- ISNUMBER() (1)
- ISO 8601 (1)
- iso Date Format (1)
- iso Format (3)
- ISO Image (3)
- isset() (1)
- IT (1)
- IT Certification (1)
- IT Exames (1)
- Itzik Ben-Gan (1)
- Japanese (1)
- Japanese Characters (1)
- Java (3)
- JavaScript (35)
- JavaScript Array (3)
- JavaScript Block (1)
- JavaScript Debug (1)
- JavaScript Download (1)
- JavaScript Event (1)
- JavaScript File (1)
- JavaScript File Download (1)
- Javascript File Generated by PHP (1)
- JavaScript Key Code (1)
- JavaScript Keycode (1)
- Javascript to PHP (1)
- JeSO (1)
- Job (2)
- Join (1)
- JS (1)
- JSON (3)
- JSON Format (1)
- Ken Stevens (2)
- Key (4)
- Key Word (1)
- Key-only Array (1)
- Keyword (2)
- Koncord (3)
- Koncord Applied Excel Functions (2)
- Koncord Cleanser (1)
- Koncord Homepage (2)
- Korean (1)
- Korean Characters (1)
- Lambda Expression (1)
- Landscape (1)
- lang_zh_Hans (1)
- lang_zh_Hant (1)
- Language (3)
- Languages (1)
- Large Array (1)
- Last Weekday (1)
- last_day (2)
- Latitude (2)
- Leap Year (1)
- Length (3)
- Line Break (1)
- Linear String (1)
- Link (2)
- Linked Server (1)
- Linux (1)
- ListBox (1)
- Literal (3)
- Loading (1)
- Local Address (1)
- Logic (1)
- Logic Bug (2)
- Logic Error (2)
- Long URL (1)
- Longitude (2)
- Loop Statement (1)
- LPAD (1)
- lr (1)
- Machine read (1)
- Macro (1)
- Macros (1)
- Marker (1)
- Match (2)
- Mathematics (1)
- Max (1)
- Max Length (3)
- Max Size (1)
- MAX() (1)
- Mean (1)
- Median (1)
- Megapixels (1)
- Memory (1)
- meta (1)
- Method (1)
- Micro (1)
- Microsoft Access (2)
- Microsoft Bug (1)
- Microsoft Excel (1)
- Microsoft Office Access (1)
- Microsoft Visual Studio 2005 (1)
- Microsoft Visual Studio 2008 (1)
- Military Time (1)
- Minute (1)
- Missing Hard Disk Space (1)
- mod_rewrite (2)
- Modular Programming (1)
- Modules (2)
- Monday (1)
- Monitor (1)
- Move (2)
- MS Access (13)
- MS Access 2000 (1)
- MS Access 2007 (1)
- Ms Excel (1)
- Multi-statement Table-Valued Function (1)
- Multidimensional Array (5)
- Multiple Email Recipients (1)
- Multiple Parameters (1)
- Multiple Recipients (1)
- Multiple-Value Parameter (1)
- multiple-value parameters (1)
- MySQL (5)
- MySQL 5.1 (1)
- MySQL Query (1)
- Name (1)
- Namespace (1)
- NaN (1)
- NCHAR (1)
- Nested Array (1)
- Nested Functions (1)
- Nested Object Namespacing (1)
- New (1)
- Newline (2)
- No Selection (1)
- non-fatal error (1)
- Normal Form (1)
- Normally Distributed Data (1)
- not a function (1)
- NOT IN (1)
- Notepad (2)
- Nothing (1)
- Nowdoc (1)
- NTEXT (1)
- Null (5)
- Number (1)
- Number 0 (1)
- Number of Elements (1)
- Numeric (2)
- Numerical Data Type (2)
- NVARCHAR (2)
- Object (3)
- Object Oriented (1)
- Object-Oriented (1)
- ODBC (2)
- OLAP (1)
- OLAP Database (1)
- OLTP (1)
- onChange (1)
- One-stroke Handwriting (1)
- onkeydown (1)
- onkeypress (1)
- Online (1)
- onload event (2)
- OO (1)
- OpenID (1)
- OpenID 1.1 (1)
- Operation must use an updateable query (1)
- Option (1)
- Option List (2)
- Optional Parameters (1)
- optionSelected (1)
- Oracle (7)
- Oracle Application Patch (1)
- Oracle Enterprise Linux (4)
- Oracle Procedure (1)
- Oracle VM (1)
- Oracle VM Template (1)
- Oracle XML Function (1)
- ORDER BY (3)
- Outer Join (1)
- Outer Query (1)
- OUTPUT (2)
- OVER PARTITION BY (1)
- Override Order (2)
- Parameter (7)
- Parameter Management (2)
- Parameter Sequence (1)
- Parameters (2)
- Parentheses () (1)
- Parse Error (1)
- Parsing Inside (1)
- PARTITION (1)
- Passing Array (1)
- Passing Function (2)
- Passing Name (1)
- Passing Reference (1)
- Passing Value (1)
- Passing Value iFrame (1)
- Paste (2)
- Paste Values (1)
- Patch (1)
- Percent (2)
- Percentage (1)
- Percentage Format (1)
- Performance (1)
- Performane Tuning (2)
- Permission (1)
- Peter Michaux (1)
- PHP (19)
- PHP Array (2)
- PHP Tag (1)
- PHP Wrapping JavaScript Debugging Method (2)
- phpinfo() (1)
- PIVOT (4)
- Pixel (1)
- PL/SQL (8)
- Portrait (1)
- Precise Radius (1)
- Prefix 'N' (1)
- Preselected (1)
- Privacy (1)
- Private (3)
- Private Search Engine (2)
- Probability Distribution (1)
- Procedure (1)
- Progress Bar (1)
- Project (1)
- Projection (1)
- Protected (1)
- Prototype (1)
- Public (3)
- public_html (1)
- Publisher ID (1)
- Pure Code Editor (1)
- push() (1)
- PV (1)
- q (1)
- Q and A (5)
- qmchenry (1)
- Radius (1)
- Random Access (1)
- Reconstruct Function (1)
- Recover (1)
- Recovery (1)
- Redirect (2)
- ref cursor (1)
- Reference (2)
- Reference Instantiate (1)
- RegExp (4)
- Regular (1)
- Regular Expression (1)
- Remote Server (1)
- Removal (1)
- Remove (1)
- Report (6)
- Reporting (1)
- Reporting Services (2)
- Reporting Services Database (1)
- ReportingServices.js (1)
- Require (1)
- Resolution (1)
- RewriteEngine (1)
- RewriteOptions (1)
- RewriteRule (1)
- Rizal Almashoor (1)
- Robotics (1)
- Ron de Bruin (1)
- ROUND() (2)
- ROUNDDOWN() (1)
- Rounding (1)
- Row (1)
- Row to Column (1)
- ROWNUM (1)
- sa (1)
- Saturday (1)
- Save As (1)
- SaveAs (1)
- Schedule (2)
- Screen Size (1)
- Script (1)
- Script File (2)
- scrollHeight (1)
- Search (2)
- Search Engine (4)
- Seasonal Adjustment (1)
- Secret Process (1)
- SELECT (4)
- Select List (1)
- SELECT PIVOT (2)
- SELECT TOP (1)
- Selected Item (1)
- selectedIndex (1)
- Selector (1)
- Self JOIN (1)
- Self-ting Temporary Function (1)
- self:: (1)
- SEO (2)
- Sequence (3)
- Sequence of Parameters (1)
- Sequence to Execute Modules (2)
- Sequential Number (1)
- Sequential Programming (1)
- Series Number (1)
- Server Virtualization (1)
- set (4)
- SET NAMES (1)
- SETI(a)home (1)
- setInterval (2)
- setTimeout (1)
- SetWarnings (1)
- Shared Server (1)
- Show/Hide (1)
- SHP (1)
- sign() (1)
- Simplified Chinese (2)
- SIZE (1)
- sizeof() (1)
- Slow Computer (1)
- Smifis (1)
- Software Engineering (1)
- Solution (1)
- Sort (1)
- Sorting (2)
- Sorting Order (1)
- SP (1)
- sp_executesql (2)
- Space (1)
- SPAN (1)
- Specific Radius (1)
- Speed (1)
- sq_addlinkedserver (1)
- sq_addlinkedsrvlogin (1)
- SQL (5)
- SQL Editor (1)
- SQL Query (1)
- SQL Server (12)
- SQL Server Agent (1)
- SQL Server Analysis Services (1)
- SQL Server Business Intelligence Development Studio (1)
- SQL Server Integration Services (1)
- SQL Server Management Studio (2)
- SQL Server Native Client 10.0 (2)
- SQL Server Reporting Services (6)
- SQL Server Reporting Services 2005 (2)
- SSAS (1)
- SSIS (1)
- SSRS (7)
- SSRS 2005 (3)
- SSRS 2008 (2)
- SSRS Parameter (1)
- Standard Deviation (2)
- Startup (1)
- Stateless (1)
- Static (2)
- Status Bar (1)
- STD() (1)
- STDDEV() (1)
- STDEVP() (1)
- Stored Procedure (7)
- String (6)
- String 'null' (1)
- String 0 (1)
- String Parse (1)
- String Reference (2)
- Stringify (1)
- stringify() (1)
- strlen() (1)
- Style Properties (1)
- subdomain (1)
- SUBSTRING (1)
- SUM() (2)
- SUM(CASE) GROUP BY Method (3)
- Summation (1)
- Summation of Hours (1)
- Sunday (1)
- Suppress (1)
- T-SQL (26)
- Tab (2)
- Table (3)
- Table Name (2)
- Table of Contents (1)
- Table Structure (1)
- Table Type (1)
- Table() (1)
- Task Manager (1)
- td (2)
- Telephone Number Parse (1)
- Temp Table (2)
- Temporary Table (2)
- Terms (1)
- Terms Of Services (1)
- Test Books (1)
- Text (1)
- The report definition is not valid (1)
- The SELECT item identified by the ORDER BY number 1 contains a variable as part of the expression identifying a (1)
- this (2)
- Thursday (1)
- Tin() (1)
- TINYINT (1)
- Tinyint() (1)
- Title (1)
- To_number() (1)
- Today() (1)
- Tool (1)
- Toolbar (1)
- TOP (1)
- TOP (n) PERCENT (1)
- Total (1)
- tr (1)
- Traditional Chinese (2)
- Transact-SQL (2)
- TRANSFORM (4)
- Transpose (4)
- trim() (1)
- TRUE (1)
- Truncate (2)
- Tuesday (1)
- Tutorial (7)
- typeof() (1)
- undefined() (1)
- UNDELETE (1)
- UNDO (1)
- Uneven Array (1)
- Unexpected $end (1)
- Unicode (2)
- Unicode 3.0 (1)
- UNION (5)
- UNION ALL (1)
- Unknown Dimentions (1)
- Unneeded Parameters (1)
- unset() (2)
- Unwanted Parameters (1)
- Update (2)
- Upload (1)
- Upload Data (1)
- Upper letters (1)
- Urban Legend (1)
- URL (1)
- URL Redirect (2)
- Usability (1)
- use Varibalized Function (1)
- UTF-16 (1)
- UTF-8 (1)
- UTF8 (1)
- Value (2)
- Value Passing (1)
- var (1)
- VARCHAR (1)
- Varchar To Integer (1)
- VARCHAR(MAX) (2)
- Variable Assignment (1)
- Variable Declaration (1)
- Variable Passing (2)
- Variablized Function (4)
- VB6 (2)
- VBA (3)
- VDS (1)
- Vertical (1)
- Virtual Dedicated Server (1)
- Virtual Private Server (1)
- Virtual URL (1)
- Visited (1)
- Visual Basic 6.0 (2)
- Visual Studio (1)
- VMware (1)
- VMware Server (2)
- VPS (1)
- WebSearch (1)
- Wednesday (1)
- Week Start Day (1)
- WEEKDAY (1)
- WEEKDAY() (2)
- WHERE (2)
- WHERE Condition (3)
- WHERE IN (1)
- WHERE NOT IN (1)
- Whitespace (2)
- WHUXGA (1)
- Width (1)
- window.innerWidth (1)
- window.onload Event (1)
- Windows Authentication (1)
- windows.event (1)
- WIP (1)
- WITH (2)
- With Parameter (1)
- Without Data (1)
- word-wrap: break-word; (1)
- www. (1)
- www2 (1)
- XML (1)
- XML cannot be the whole program (1)
- XML Tag (1)
- Year-To-Date (1)
- ZEROFILL (1)
- zh-Hans (1)
- zh-Hant (1)
- σ (1)